创建Maven项目
查看博客:idea创建Maven项目
引入依赖
1 2 3 4 5 6 7 8 9 10 11 12 13
| <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.2.9.RELEASE</version> </dependency>
<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.20</version> <scope>provided</scope> </dependency>
|
测试
配置文件
命名为:applicationContext.xml,完整代码如下:
1 2 3 4 5 6 7 8 9 10 11
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student1" class="com.ledao.entity.Student"> <property name="id" value="1"/> <property name="name" value="张三"/> <property name="age" value="11"/> </bean> </beans>
|
学生实体类
命名为:Student.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.ledao.entity;
import lombok.Data;
@Data public class Student {
private Integer id;
private String name;
private Integer age; }
|
测试类
命名为:Test.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.ledao;
import com.ledao.entity.Student; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) { ApplicationContext context=new ClassPathXmlApplicationContext("applicationContext.xml"); Student student = (Student) context.getBean("student1"); System.out.println(student.getId()+","+student.getName()+","+ student.getAge()); } }
|
结果
运行测试类的main方法,结果如下:
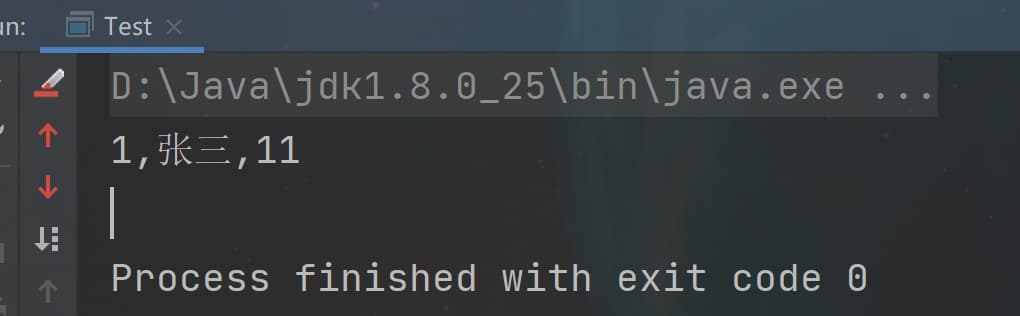