概述
通过设置Docket,还可以配置很多功能,比如是否开启swagger,过滤,分组等
开启和关闭swagger
开发环境开启swagger,生产环境就关闭swagger
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package com.ledao.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.util.ArrayList;
@Configuration public class SwaggerConfig {
@Bean public Docket createRestApi() { return new Docket(DocumentationType.OAS_30) .enable(true); } }
|
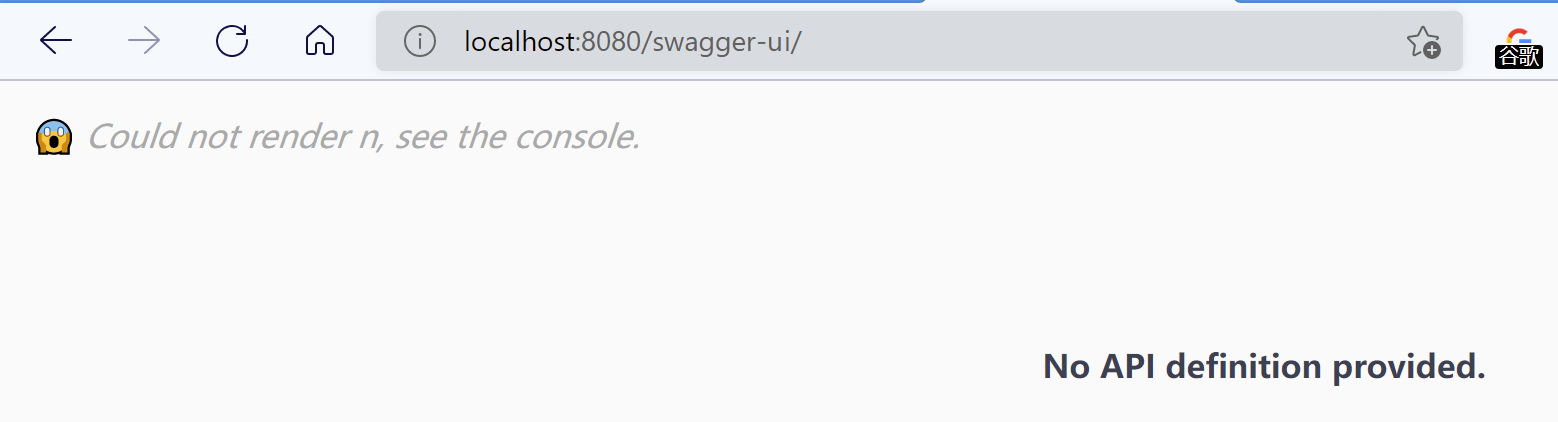
过滤
过滤方式有两种:①根据包路径过滤 ②根据请求路径过滤,这两种过滤方式可以单独使用,也可以结合使用
根据包路径
先调用select方法,然后调用apis方法,最后调用build方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| package com.ledao.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.util.ArrayList;
@Configuration public class SwaggerConfig {
@Bean public Docket createRestApi() { return new Docket(DocumentationType.OAS_30) .enable(true) .select() .apis(RequestHandlerSelectors.basePackage("com.ledao.controller")) .build(); } }
|
根据请求路径
先调用select方法,然后调用apis方法,最后调用build方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| package com.ledao.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.util.ArrayList;
@Configuration public class SwaggerConfig {
@Bean public Docket createRestApi() { return new Docket(DocumentationType.OAS_30) .enable(true) .select() .paths(PathSelectors.ant("/student/**")) .build(); } }
|
结合使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| package com.ledao.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.util.ArrayList;
@Configuration public class SwaggerConfig {
@Bean public Docket createRestApi() { return new Docket(DocumentationType.OAS_30) .enable(true) .select() .apis(RequestHandlerSelectors.basePackage("com.ledao.controller")) .paths(PathSelectors.ant("/student/**")) .build(); } }
|
分组
在实际项目开发中,把复杂项目划分多模块给多个小组或者多个人负责开发,所以每个小组或者个人要实现自己的分组,方便查找到API接口开发负责人,沟通和处理问题
通过.groupName设置分组名称,有多少个分组就搞几份配置,下面代码设置了两个分组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91
| package com.ledao.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.util.ArrayList;
@Configuration public class SwaggerConfig {
@Bean public Docket createRestApi() { return new Docket(DocumentationType.OAS_30) .groupName("开发组001") .enable(true) .select() .apis(RequestHandlerSelectors.basePackage("com.ledao.controller")) .paths(PathSelectors.ant("/student/**")) .build() .apiInfo(createApiInfo()); }
@Bean public ApiInfo createApiInfo() { return new ApiInfo( "LeDao Swagger3", "LeDao Api文档", "3.0", "https://blog.zoutl.cn", new Contact("LeDao", "https://blog.zoutl.cn", "f110@gmail.com"), "Apache 2.0", "https://www.apache.org/licenses/LICENSE-2.0", new ArrayList<>() ); }
@Bean public Docket createRestApi2() { return new Docket(DocumentationType.OAS_30) .groupName("开发组002") .enable(true) .select() .apis(RequestHandlerSelectors.basePackage("com.ledao.test")) .paths(PathSelectors.ant("/teacher/**")) .build() .apiInfo(createApiInfo2()); }
@Bean public ApiInfo createApiInfo2() { return new ApiInfo( "乐道 Swagger3", "乐道 Api文档", "3.0", "https://blog.zoutl.cn", new Contact("乐道", "https://blog.zoutl.cn", "f119@gmail.com"), "Apache 2.0", "https://www.apache.org/licenses/LICENSE-2.0", new ArrayList<>() ); } }
|