概述
关于HashMap键排序,如果是升序排序可以直接使用TreeMap,降序排序则需要自己实现
关于HashMap值排序,无论是升序还是降序,都需要自己实现,和键降序排序实现方式大同小异
下面只实现键降序排序和值排序,不实现键升序排序,因为键的升序排序直接用TreeMap就可以了
代码实现
键降序排序
实现原理
将HashMap中的EntrySet取出放入一个ArrayList中,来对ArrayList中的EntrySet进行排序,从而实现对HashMap的值进行排序的效果
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| import java.util.*;
public class Test {
public static void main(String[] args) { Map<Character, Integer> hashMap = new HashMap<>(16); hashMap.put('e', 2); hashMap.put('d', 1); hashMap.put('v', 6); hashMap.put('b', 3); hashMap.put('a', 3); List<Map.Entry<Character, Integer>> list = new ArrayList<>(hashMap.entrySet()); Collections.sort(list, new Comparator<Map.Entry<Character, Integer>>() { @Override public int compare(Map.Entry<Character, Integer> o1, Map.Entry<Character, Integer> o2) { return o2.getKey() - o1.getKey(); } }); for (Map.Entry<Character, Integer> entry : list) { System.out.println(entry.getKey() + ": " + entry.getValue()); } } }
|
值排序
实现原理
同上,区别如下图:
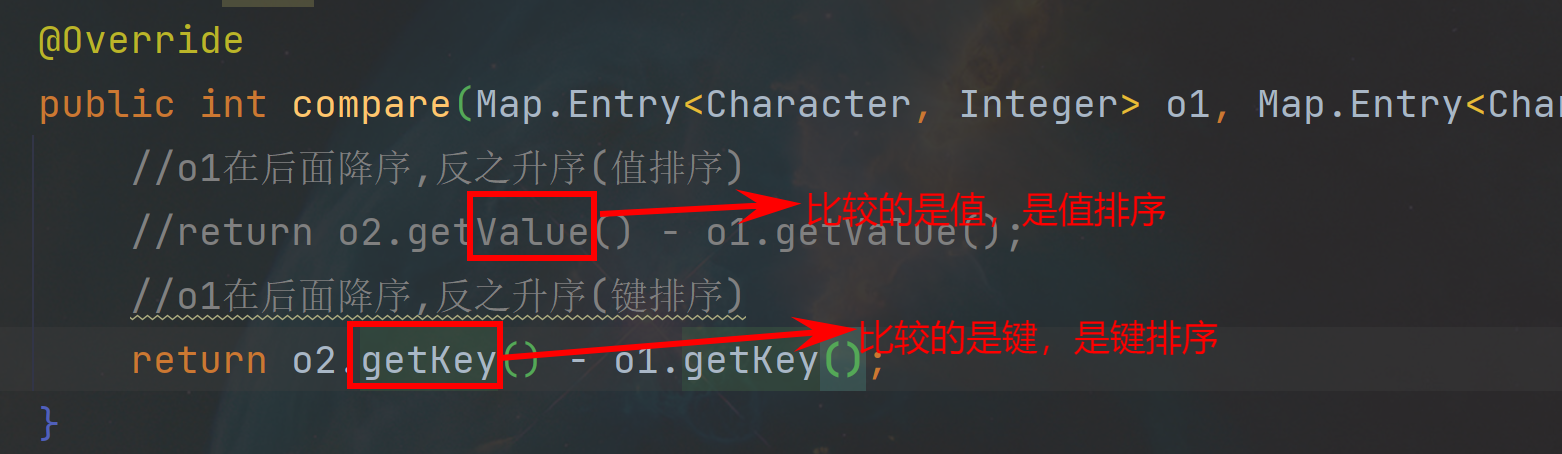
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| import java.util.*;
public class Test {
public static void main(String[] args) { Map<Character, Integer> hashMap = new HashMap<>(16); hashMap.put('e', 2); hashMap.put('d', 1); hashMap.put('v', 6); hashMap.put('b', 3); hashMap.put('a', 9); List<Map.Entry<Character, Integer>> list = new ArrayList<>(hashMap.entrySet()); Collections.sort(list, new Comparator<Map.Entry<Character, Integer>>() { @Override public int compare(Map.Entry<Character, Integer> o1, Map.Entry<Character, Integer> o2) { return o2.getValue() - o1.getValue(); } }); for (Map.Entry<Character, Integer> entry : list) { System.out.println(entry.getKey() + ": " + entry.getValue()); } } }
|