项目结构
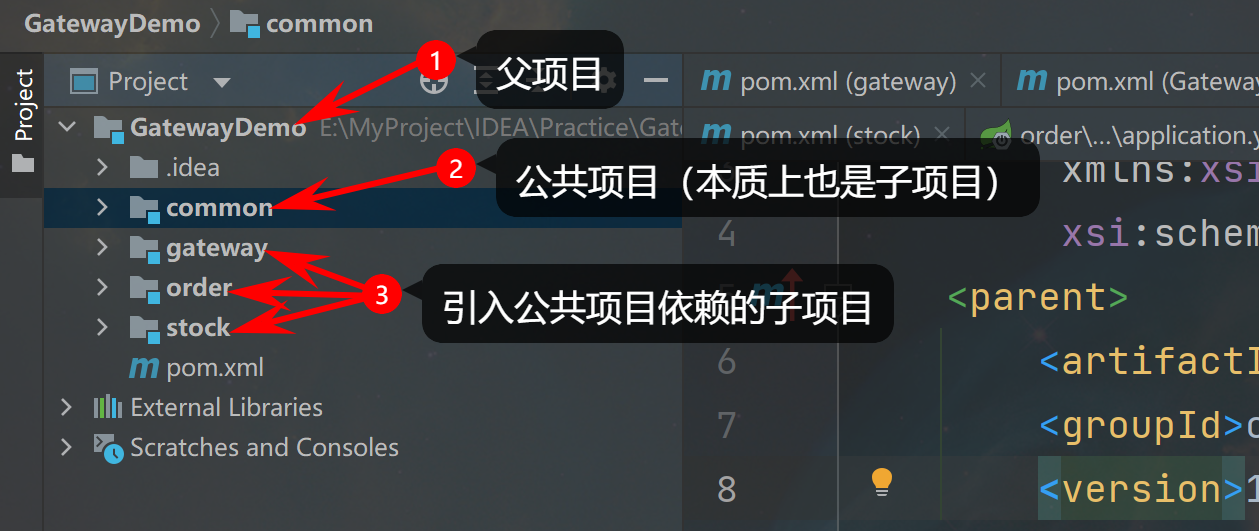
父项目
创建一个父项目(Maven项目),只用来管理依赖的版本
首先将packaging
节点的内容设置为pom,表明只是一个pom项目,只用来聚合工程或传递依赖
1
| <packaging>pom</packaging>
|
在properties
节点内管理依赖的版本
1 2 3 4 5 6 7 8 9
| <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <spring-cloud.version>Hoxton.SR9</spring-cloud.version> <springboot.version>2.3.2.RELEASE</springboot.version> <springcloudalibaba.version>2.2.6.RELEASE</springcloudalibaba.version> <gson.version>2.9.0</gson.version> </properties>
|
在dependencyManagement
节点内定义依赖,然后这些依赖可以在子项目中引用了
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>${springboot.version}</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-alibaba-dependencies</artifactId> <version>${springcloudalibaba.version}</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>${gson.version}</version> </dependency> </dependencies> </dependencyManagement>
|
在子项目中引用的格式如下:(以上面定义的Gson依赖为例,在下面的代码中可以看到省略了版本号
)
1 2 3 4
| <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> </dependency>
|
公共项目
创建一个公共项目(Maven项目,是父项目的子项目),管理公共依赖、工具类以及实体类等
创建好这个公共项目后,idea在父项目的pom.xml
文件的modules
节点就引入了这个公共模块(每创建一个子项目就会自动在父项目中引入)
1 2 3
| <modules> <module>common</module> </modules>
|
在dependencies
节点中引入公共依赖,就是大多数子项目(两个及以上)都可能会用到的依赖,如果只有某一个子项目单独使用的依赖,就直接在那个子项目pom.xml
文件的dependencies
节点引入(已经被父项目管理的依赖可以省略版本号,建议省略掉,直接用父项目管理版本号即可)
1 2 3 4 5 6
| <dependencies> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> </dependency> </dependencies>
|
子项目
创建子项目(Maven项目,真实的项目),引入公共项目的依赖,格式如下:
1 2 3 4 5 6 7
| <dependencies> <dependency> <groupId>com.ledao</groupId> <artifactId>common</artifactId> <version>1.0-SNAPSHOT</version> </dependency> </dependencies>
|
一些属性来源如下图:(在公共项目的pom.xml
中)
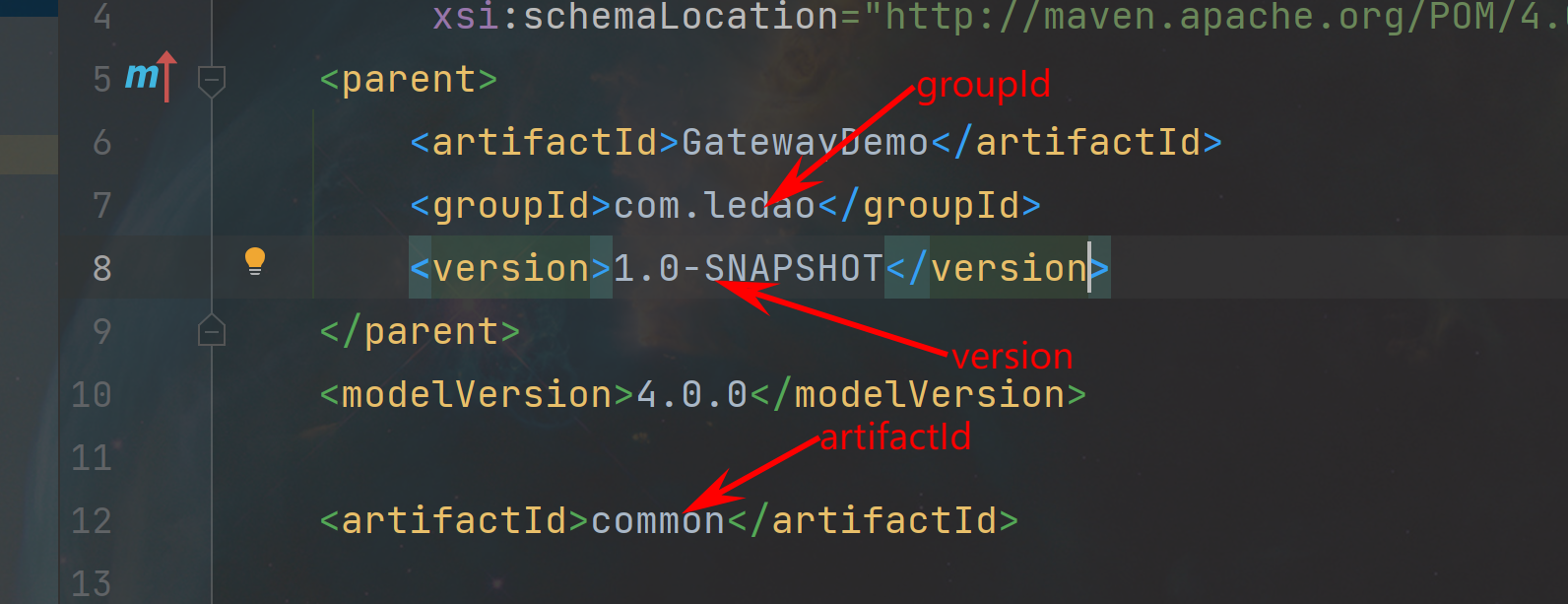
不看公共项目的pom.xml
快速引入看下面动图:(直接输入公共项目的名称就会出提示)
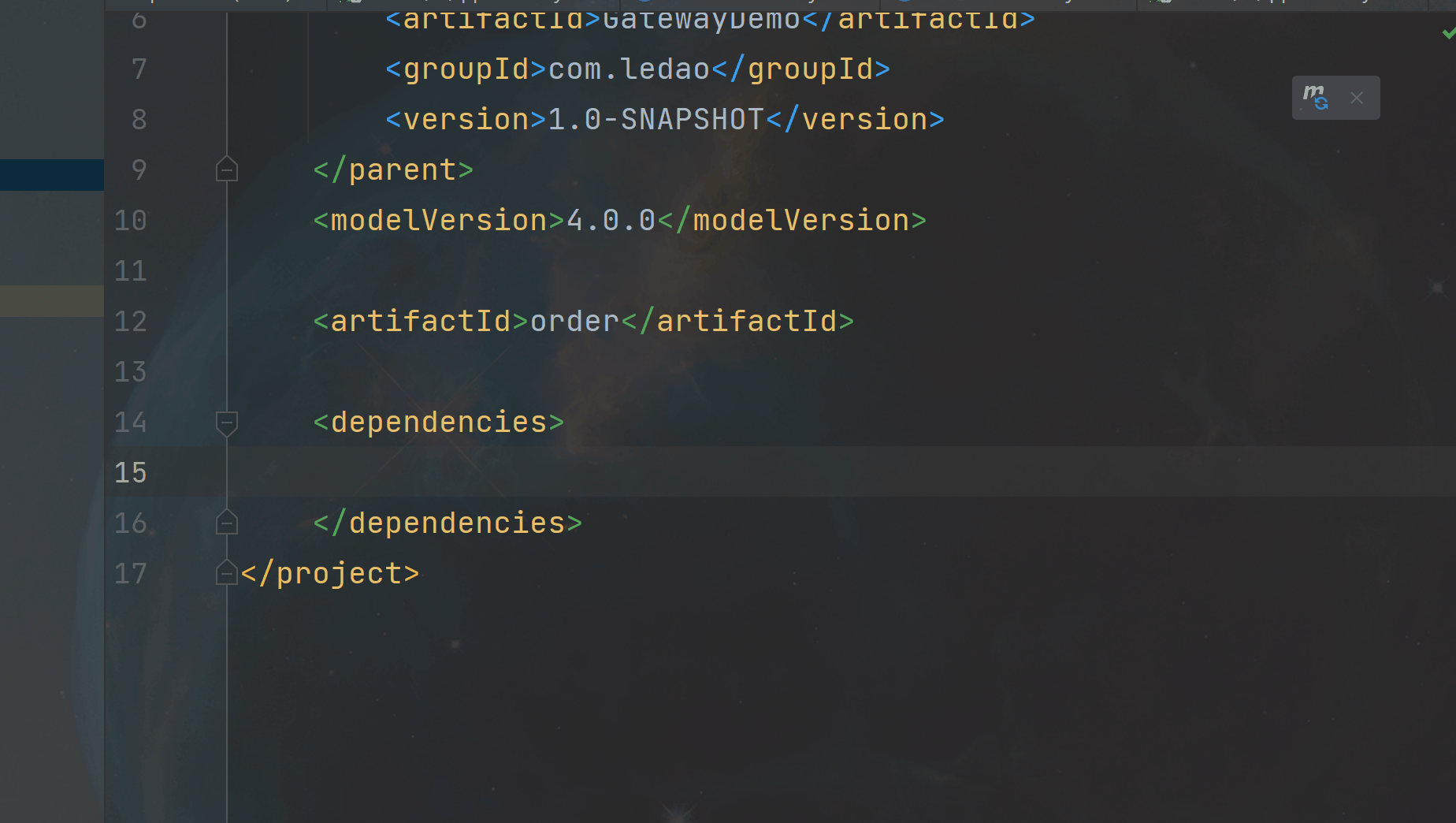
如果是在公共项目中没有引入的依赖,直接在子项目中引入即可(像上面公共项目那样引入即可)
PS.
公共项目的版本号也可以丢给父项目管理,首先在父项目的properties
节点定义版本号
1
| <common.version>1.0-SNAPSHOT</common.version>
|
在dependencyManagement.dependencies
节点中引入
1 2 3 4 5
| <dependency> <groupId>com.ledao</groupId> <artifactId>common</artifactId> <version>${common.version}</version> </dependency>
|
在子项目中引入,和上面对比少了版本号
1 2 3 4 5 6
| <dependencies> <dependency> <groupId>com.ledao</groupId> <artifactId>common</artifactId> </dependency> </dependencies>
|