JWT介绍
关于JWT的详细介绍可以查看博客:JSON Web Token 入门教程 - 阮一峰的网络日志 (ruanyifeng.com)
或者直接去官网了解(官网可直接解码JWT):JSON Web Tokens - jwt.io
代码实现
引入依赖
该依赖的接口文档网址为:JWT - java-jwt 4.2.1 javadoc
1 2 3 4 5 6
| <dependency> <groupId>com.auth0</groupId> <artifactId>java-jwt</artifactId> <version>4.2.1</version> </dependency>
|
生成和验证
我把JWT的生成和验证封装成了一个工具类,以方便调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116
| package com.ledao.util;
import com.auth0.jwt.JWT; import com.auth0.jwt.JWTVerifier; import com.auth0.jwt.algorithms.Algorithm; import com.auth0.jwt.exceptions.SignatureVerificationException; import com.auth0.jwt.exceptions.TokenExpiredException; import com.auth0.jwt.interfaces.DecodedJWT;
import java.util.Date; import java.util.UUID;
public class JwtUtil {
private static final int TIME = 1000 * 60 * 60 * 2;
private static final String TOKEN_SECRET = "ledao";
public static String createToken(String roleName) { return JWT.create() .withJWTId(UUID.randomUUID().toString()) .withIssuer("乐道") .withIssuedAt(new Date(System.currentTimeMillis())) .withExpiresAt(new Date(System.currentTimeMillis() + TIME)) .withAudience(roleName) .withSubject("mall-admin") .sign(Algorithm.HMAC512(TOKEN_SECRET)); }
public static boolean checkToken(String token) { if (token == null) { return false; } try { JWTVerifier jwtVerifier = JWT.require(Algorithm.HMAC512(TOKEN_SECRET)).build(); jwtVerifier.verify(token); } catch (SignatureVerificationException e) { return false; } catch (TokenExpiredException e) { return false; } catch (Exception e) { System.out.println(e.getMessage()); return false; } return true; }
public static DecodedJWT decodedJWT(String token) { return JWT.decode(token); }
public static void main(String[] args) { String token = createToken("admin"); System.out.println(token); System.out.println("验证结果: " + checkToken(token)); System.out.println("编号: " + decodedJWT(token).getId()); System.out.println("签发时间: " + decodedJWT(token).getIssuedAt()); System.out.println("过期时间: " + decodedJWT(token).getExpiresAt()); System.out.println("签发人: " + decodedJWT(token).getIssuer()); System.out.println("所有受众: " + decodedJWT(token).getAudience()); System.out.println("受众1: " + decodedJWT(token).getAudience().get(0)); } }
|
结果截图
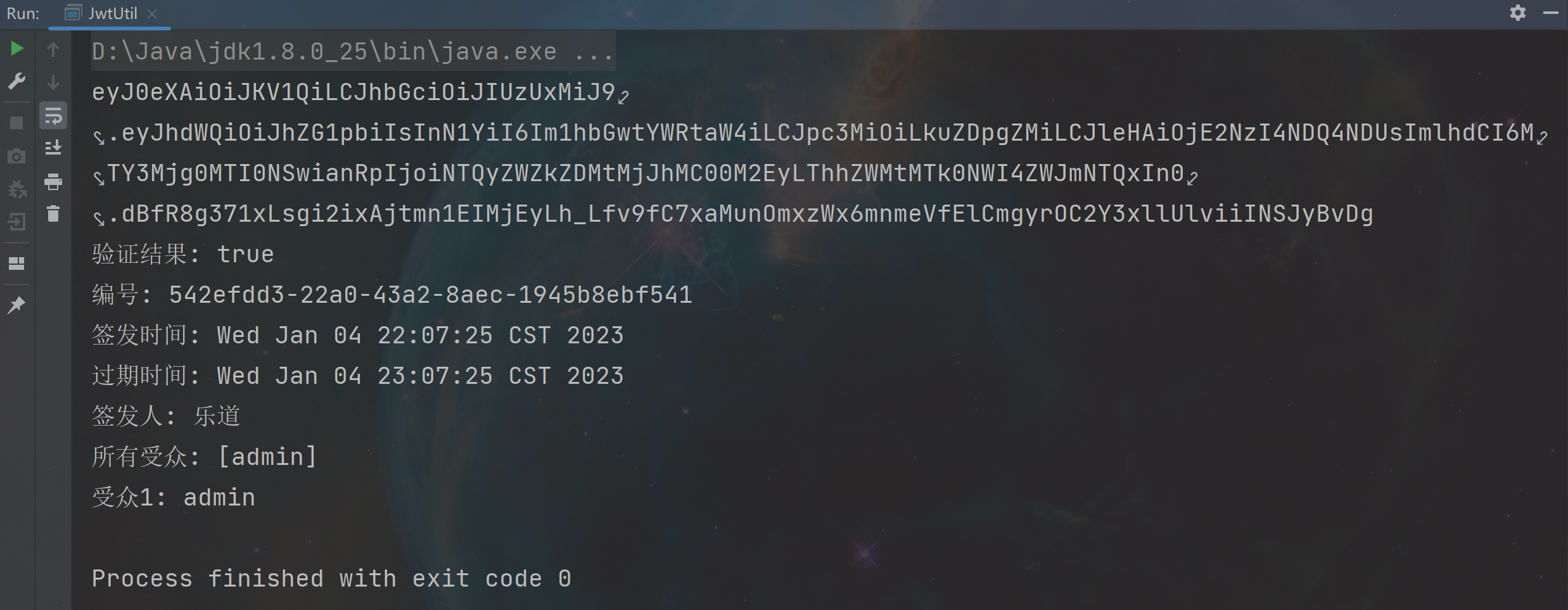
关于续约
可以观看视频:【IT老齐251】无状态的JWT令牌如何实现自动续约_哔哩哔哩_bilibili