介绍
和使用浏览器的全文翻译(机器翻译)相比,使用i18n实现国际化(人工翻译)应该会更准确,但是挺麻烦的,因为每一个组件的文字都需要翻译和配置
实现过程
安装
1
| npm install vue-i18n@next
|
新建语言配置文件
在src目录下新建一个名为locales
的目录,在这个目录下新建一个名为lang
的目录、i18n.js
和index.js
文件,最后在lang
目录下新建en.js
和zh.js
文件
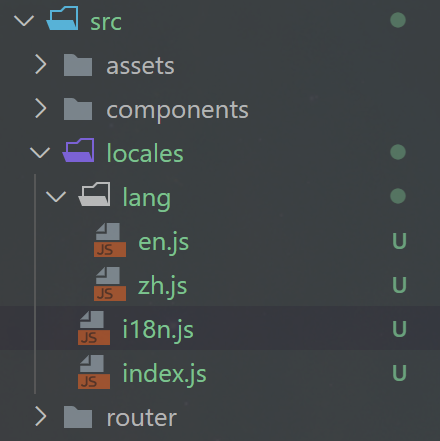
en.js
的内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| export default { menu: { name: 'Background', home: 'Home', userManage: 'Manage User', announcementManage: 'Manage Notice', typeManage: 'Manage Type', bigTypeManage: 'Manage BigType', smallTypeManage: 'Manage SmallType', goodsManage: 'Manage Goods', orderManage: 'Manage Order', valuationManage: 'Manage Valuation' }, main: { home: 'Home', full: 'Full Screen', noFull: 'Exit Full Screen', openMenu: 'Open The Menu', closeMenu: 'Close The Menu', modifyPassword: 'Modify Password', logout: 'Logout', closeAll: 'Close All', closeLeft: 'Close The Left', closeRight: 'Close The Right' } }
|
zh.js
的内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| export default{ menu:{ name:'后台管理系统模板', home:'首页', userManage:'用户管理', announcementManage: '公告管理', typeManage: '商品分类管理', bigTypeManage: '商品大类', smallTypeManage: '商品小类', goodsManage: '商品管理', orderManage: '订单管理', valuationManage: '评价管理' }, main:{ home:'首页', full: '全屏', noFull: '退出全屏', openMenu:'打开菜单', closeMenu:'关闭菜单', modifyPassword: '修改密码', logout: '注销', closeAll: '关闭全部', closeLeft: '关闭左侧', closeRight: '关闭右侧' } }
|
index.js
的内容如下:
1 2 3 4 5 6 7
| import en from './lang/en' import zh from './lang/zh'
export default { en, zh, }
|
i18n.js
的内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import { createI18n } from 'vue-i18n' import messages from './index' const language = ( (navigator.language ? navigator.language : navigator.userLanguage) || "zh" ).toLowerCase(); const i18n = createI18n({ fallbackLocale: 'zh', globalInjection: true, legacy: false, locale: language.split("-")[0] || "zh", messages, });
export default i18n
|
引入
在main.js
中引入
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import { createApp } from 'vue' import { createPinia } from "pinia" import piniaPluginPersistedstate from "pinia-plugin-persistedstate"; import './style.css' import App from './App.vue'
import router from './router' import i18n from './locales/i18n';
const pinia = createPinia() pinia.use(piniaPluginPersistedstate)
const app = createApp(App) app.use(pinia).use(router).use(i18n).mount('#app')
|
使用
在要实现国际化的页面使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <template> <div> <p>{{ t('main.home') }}</p> </div> </template>
<script setup> import { ref, getCurrentInstance } from "vue"; import { useI18n } from 'vue-i18n'
const { proxy } = getCurrentInstance();
function change(type) { proxy.$i18n.locale = type; } const { t } = useI18n() </script>
<style scoped>
</style>
|