引入依赖
1 2 3 4 5 6
| <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.10</version> </dependency>
|
使用的方法
JsonElement parseString(String json)
解析json字符串为JsonElement
JsonObject getAsJsonObject()
将字符串解析为JsonObject对象
JsonObject getAsJsonObject(String memberName)
获取某个对象
JsonElement get(String memberName)
获取对象的属性值
String getAsString()
返回对象属性值的字符串值
测试
要测试的JSON字符串
这次测试是获取data.token.accessToken
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| { "code": 2000, "msg": null, "data": { "uuid": null, "username": null, "nickname": null, "avatar": null, "blog": null, "company": null, "location": null, "email": null, "remark": null, "gender": "UNKNOWN", "source": "GITHUB", "token": { "accessToken": "gho_V7z5mRl3zrZAclRnSdhmczkYW2jvx", "expireIn": 0, "refreshToken": null, "uid": null, "openId": null, "accessCode": null, "unionId": null, "scope": null, "tokenType": "bearer", "idToken": null, "macAlgorithm": null, "macKey": null, "code": null, "oauthToken": null, "oauthTokenSecret": null, "userId": null, "screenName": null, "oauthCallbackConfirmed": null } } }
|
Java代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| import com.google.gson.JsonElement; import com.google.gson.JsonObject; import com.google.gson.JsonParser;
public class Test {
public static void main(String[] args) { String json = "{\n" + " \"code\": 2000,\n" + " \"msg\": null,\n" + " \"data\": {\n" + " \"uuid\": null,\n" + " \"username\": null,\n" + " \"nickname\": null,\n" + " \"avatar\": null,\n" + " \"blog\": null,\n" + " \"company\": null,\n" + " \"location\": null,\n" + " \"email\": null,\n" + " \"remark\": null,\n" + " \"gender\": \"UNKNOWN\",\n" + " \"source\": \"GITHUB\",\n" + " \"token\": {\n" + " \"accessToken\": \"gho_V7z5mRl3zrZAclRnSdhmczkYW2jvx\",\n" + " \"expireIn\": 0,\n" + " \"refreshToken\": null,\n" + " \"uid\": null,\n" + " \"openId\": null,\n" + " \"accessCode\": null,\n" + " \"unionId\": null,\n" + " \"scope\": null,\n" + " \"tokenType\": \"bearer\",\n" + " \"idToken\": null,\n" + " \"macAlgorithm\": null,\n" + " \"macKey\": null,\n" + " \"code\": null,\n" + " \"oauthToken\": null,\n" + " \"oauthTokenSecret\": null,\n" + " \"userId\": null,\n" + " \"screenName\": null,\n" + " \"oauthCallbackConfirmed\": null\n" + " }\n" + " }\n" + "}"; JsonElement jsonElement = JsonParser.parseString(json); JsonObject root = jsonElement.getAsJsonObject(); JsonObject data = root.getAsJsonObject("data"); JsonObject token = data.getAsJsonObject("token"); String accessToken = token.get("accessToken").getAsString(); System.out.println(accessToken); } }
|
结果
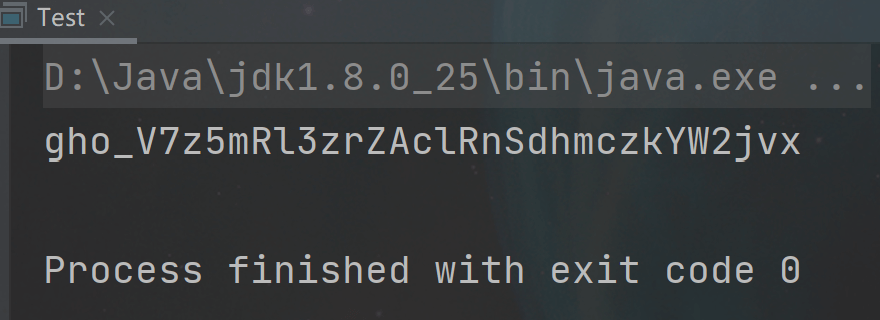