GitHub创建第三方应用
具体步骤查看博客:GitHub创建第三方应用
copy以下三个信息:Client ID、Client Secret和Authorization callback URL
application.yml配置
1 2 3 4 5 6 7 8 9 10
| server: port: 80 servlet: context-path: / tomcat: uri-encoding: utf-8
spring: session: store-type: none
|
引入依赖
1 2 3 4 5
| <dependency> <groupId>me.zhyd.oauth</groupId> <artifactId>JustAuth</artifactId> <version>1.16.1</version> </dependency>
|
创建Request
1 2 3 4 5
| AuthRequest authRequest = new AuthGithubRequest(AuthConfig.builder() .clientId("Client ID") .clientSecret("Client Secret") .redirectUri("应用回调地址") .build());
|
生成授权地址
1
| String authorizeUrl = authRequest.authorize(AuthStateUtils.createState());
|
完整代码
IndexController
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.ledao.controller;
import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class IndexController {
@RequestMapping("/") public String root() { return "<h2 style='text-align: center'><a href='/oauth/login/github'>github登录</a> <a href='/oauth/login/gitee'>gitee登录</a></span>"; }
@RequestMapping("/home") public String home() { return "<h1>主页</h1>"; } }
|
JustAuthController
Client ID、Client Secret和Authorization callback URL更换为自己的,下面代码中的是错误的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87
| package com.ledao.controller;
import me.zhyd.oauth.config.AuthConfig; import me.zhyd.oauth.model.AuthCallback; import me.zhyd.oauth.model.AuthResponse; import me.zhyd.oauth.request.AuthGiteeRequest; import me.zhyd.oauth.request.AuthGithubRequest; import me.zhyd.oauth.request.AuthRequest; import me.zhyd.oauth.utils.AuthStateUtils; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletResponse; import java.io.IOException;
@RestController @RequestMapping("/oauth") public class JustAuthController {
@RequestMapping("/login/{type}") public void login(@PathVariable String type, HttpServletResponse response) throws IOException { AuthRequest authRequest = getAuthRequest(type); response.sendRedirect(authRequest.authorize(AuthStateUtils.createState())); }
@RequestMapping("/callback/{type}") public AuthResponse callback(@PathVariable String type, AuthCallback callback) { AuthRequest authRequest = getAuthRequest(type); AuthResponse authResponse = authRequest.login(callback); return authResponse; }
private AuthRequest getAuthRequest(String type) { AuthRequest authRequest = null; switch (type) { case "github": authRequest = new AuthGithubRequest(AuthConfig.builder() .clientId("803de7712b9908181") .clientSecret("e863222b3de13fe5ca91224e4651c0316e") .redirectUri("http://localhost:80/oauth/callback/github") .build()); break; case "gitee": authRequest = new AuthGiteeRequest(AuthConfig.builder() .clientId("e7c5c4c0cadb936b728b5d87af2ae1f2958c0a2cb24af4daa6f29a5f7") .clientSecret("823a0fd650add2468a8e31ac684b7c614b1783331c73b32bb29040") .redirectUri("http://localhost:80/oauth/callback/gitee") .build()); break; default: break; } return authRequest; } }
|
结果
浏览器地址栏输入:http://localhost
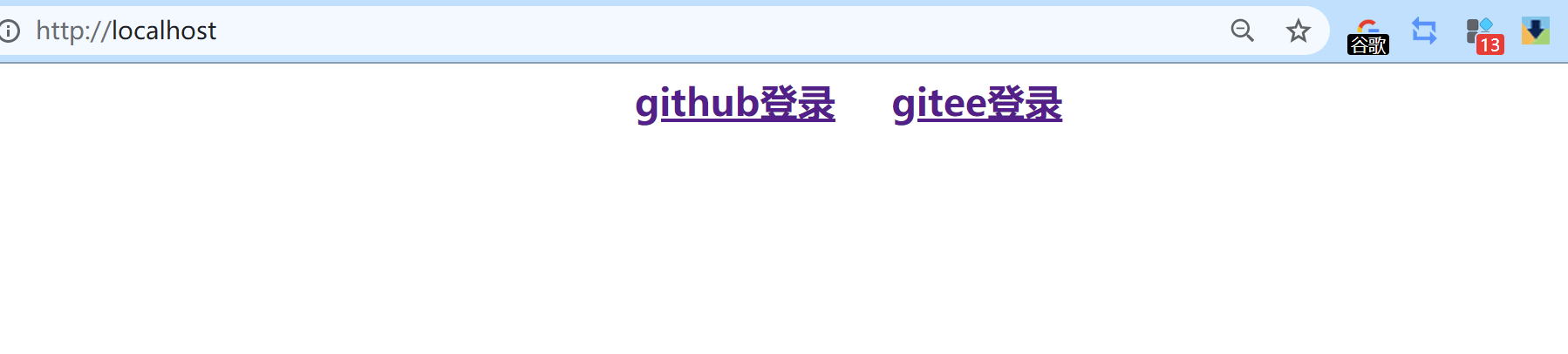
GitHub登录返回的结果
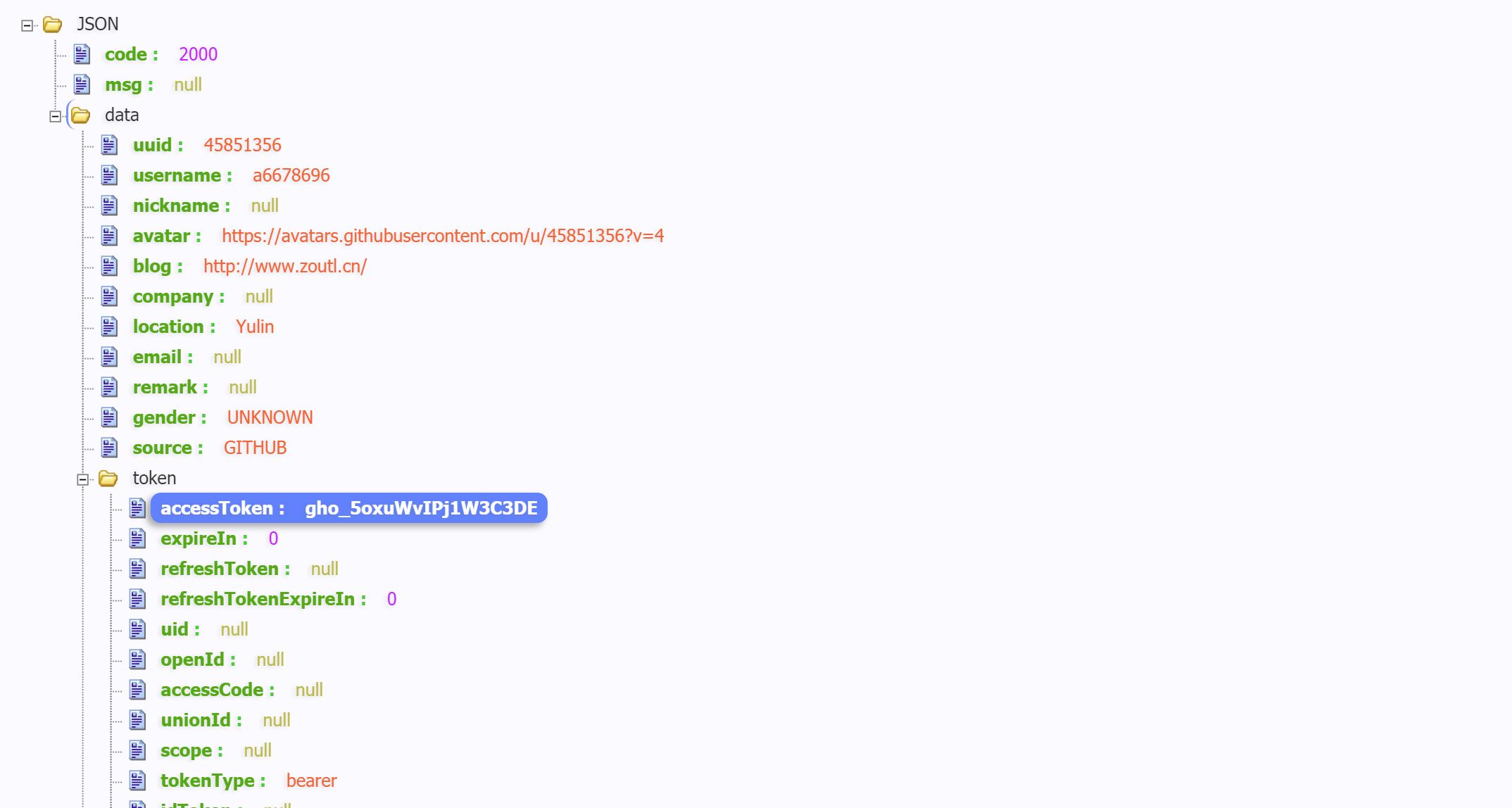
Gitee登录返回的结果
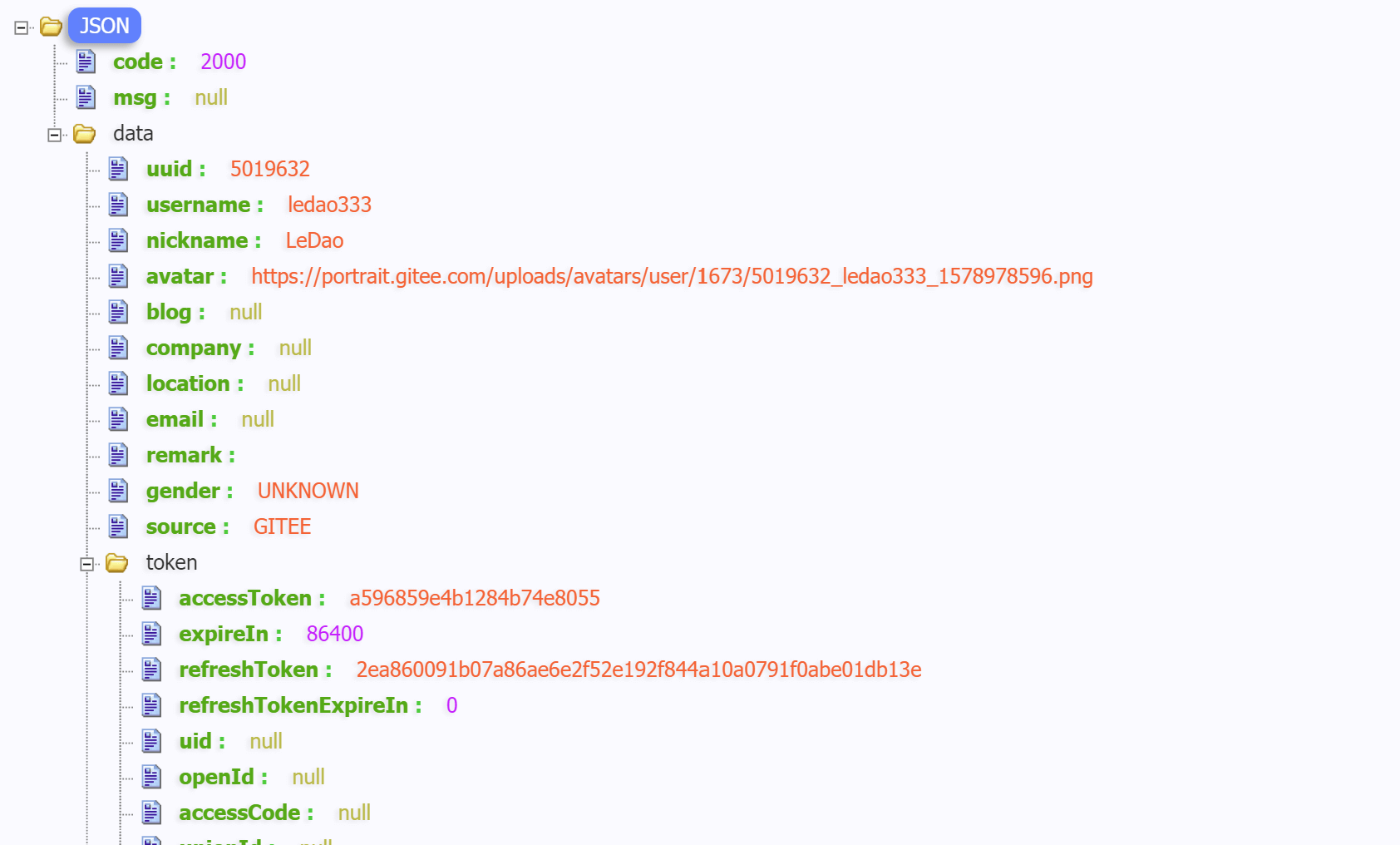
PS.
代码中包含了Gitee登录
GitHub完整代码地址:https://github.com/a6678696/JustAuthDemo
更多第三方登录教程查看:https://justauth.wiki/
如果需要获取返回的用户信息,callback方法返回的数据类型修改为AuthResponse<AuthUser>,完整代码如下:(可直接覆盖上面代码)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
|
@RequestMapping("/callback/{type}") public AuthResponse<AuthUser> callback(@PathVariable String type, AuthCallback callback) { AuthRequest authRequest = getAuthRequest(type); AuthResponse<AuthUser> authResponse = authRequest.login(callback);
System.out.println("状态码:" + authResponse.getCode());
System.out.println("用户的uuid:" + authResponse.getData().getUuid()); System.out.println("用户的昵称:" + authResponse.getData().getNickname()); System.out.println("用户的头像:" + authResponse.getData().getAvatar());
System.out.println("access_token:" + authResponse.getData().getToken().getAccessToken()); return authResponse; }
|