引入AOP的依赖
1 2 3 4 5 6
| <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.4</version> </dependency>
|
Service接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package com.ledao.service;
public interface StudentService {
void add();
void delete();
void update();
void findById(); }
|
Service接口实现类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package com.ledao.service.impl;
import com.ledao.service.StudentService;
public class StudentServiceImpl implements StudentService {
@Override public void add() { System.out.println("添加学生"); }
@Override public void delete() { System.out.println("删除学生"); }
@Override public void update() { System.out.println("修改学生"); }
@Override public void findById() { System.out.println("根据id查找学生"); } }
|
定义增强类
Log前置增强类
执行业务代码前先执行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.ledao.log;
import org.springframework.aop.MethodBeforeAdvice;
import java.lang.reflect.Method;
public class BeforeLog implements MethodBeforeAdvice {
@Override public void before(Method method, Object[] args, Object target) throws Throwable { if (target != null) { System.out.println("[BeforeLog]" + target.getClass().getName() + "下的" + method.getName() + "方法被执行了"); } } }
|
Log后置增强类
执行业务代码后再执行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.ledao.log;
import org.springframework.aop.AfterReturningAdvice;
import java.lang.reflect.Method;
public class AfterLog implements AfterReturningAdvice {
@Override public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable { System.out.println("[AfterLog]执行了" + method.getName() + "方法,返回结果为:" + returnValue); } }
|
配置文件ApplicationContext.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="studentService" class="com.ledao.service.impl.StudentServiceImpl"/> <bean id="beforeLog" class="com.ledao.log.BeforeLog"/> <bean id="afterLog" class="com.ledao.log.AfterLog"/>
<aop:config> <aop:pointcut id="pointcut" expression="execution(* com.ledao.service.impl.StudentServiceImpl.*(..))"/> <aop:advisor advice-ref="beforeLog" pointcut-ref="pointcut"/> <aop:advisor advice-ref="afterLog" pointcut-ref="pointcut"/> </aop:config> </beans>
|
测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import com.ledao.service.StudentService; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("ApplicationContext.xml"); StudentService studentService = context.getBean("studentService", StudentService.class); studentService.add(); } }
|
结果截图
添加学生的业务前后分别执行了BeforeLog和AfterLog
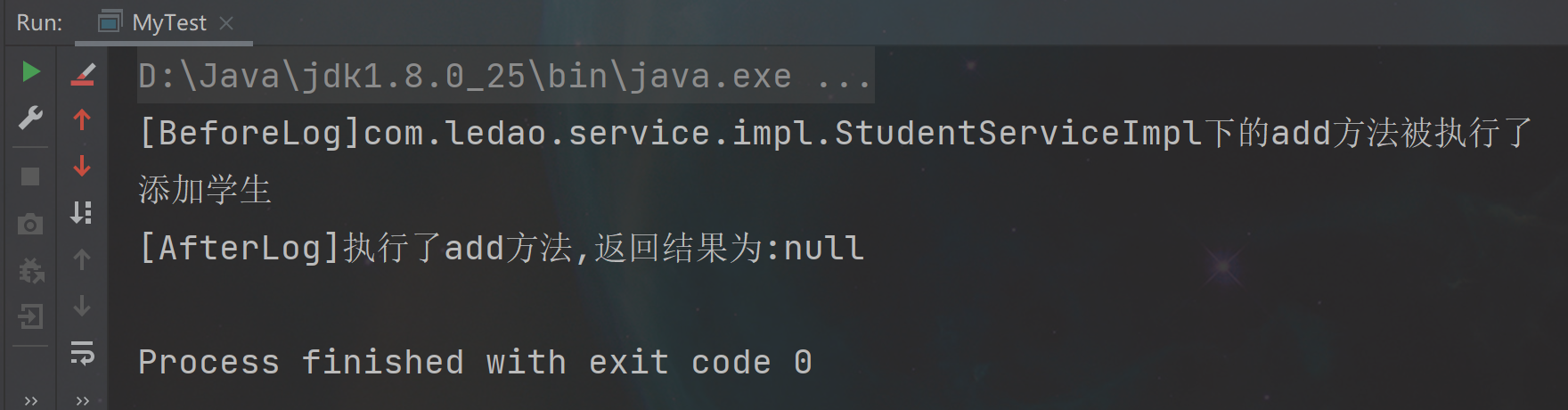