使用到的注解
@Aspect
用于标注使用这个注解的类是一个切面
@Before
执行业务代码前先执行这个方法
@After
执行业务代码后再执行这个方法
@Around
环绕增强方法使用
实现过程
Service接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package com.ledao.service;
public interface StudentService {
void add();
void delete();
void update();
void findById(); }
|
Service接口实现类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package com.ledao.service.impl;
import com.ledao.service.StudentService;
public class StudentServiceImpl implements StudentService {
@Override public void add() { System.out.println("添加学生"); }
@Override public void delete() { System.out.println("删除学生"); }
@Override public void update() { System.out.println("修改学生"); }
@Override public void findById() { System.out.println("根据id查找学生"); } }
|
AnnotationPointCut增强类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| package com.ledao.diy;
import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.Signature; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before;
@Aspect public class AnnotationPointCut {
@Before("execution(* com.ledao.service.impl.StudentServiceImpl.*(..))") public void before() { System.out.println("---------执行方法前---------"); }
@After("execution(* com.ledao.service.impl.StudentServiceImpl.*(..))") public void after() { System.out.println("---------执行方法后---------"); }
@Around("execution(* com.ledao.service.impl.StudentServiceImpl.*(..))") public void around(ProceedingJoinPoint proceedingJoinPoint) throws Throwable { System.out.println("环绕前"); Signature signature = proceedingJoinPoint.getSignature(); System.out.println("执行的业务方法为:" + signature); proceedingJoinPoint.proceed(); System.out.println("环绕后"); } }
|
配置文件ApplicationContext.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="studentService" class="com.ledao.service.impl.StudentServiceImpl"/> <bean id="annotationPointCut" class="com.ledao.diy.AnnotationPointCut"/>
<aop:aspectj-autoproxy/> </beans>
|
测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import com.ledao.service.StudentService; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("ApplicationContext.xml"); StudentService studentService = context.getBean("studentService", StudentService.class); studentService.add(); } }
|
结果截图
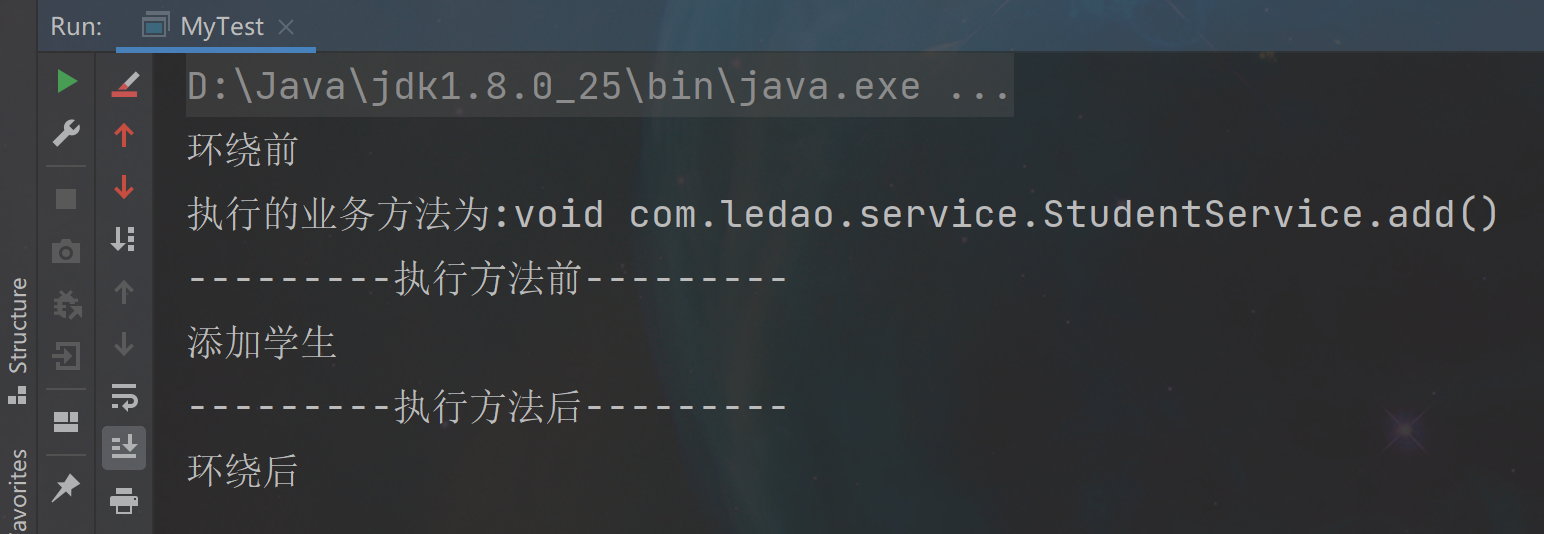
PS.
如果不想使用配置文件,则需要在配置类上使用@EnableAspectJAutoProxy
注解,这个注解的作用是:启动AspectJ自动代理,配置类代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| package com.ledao.config;
import com.ledao.dao.UserDao; import com.ledao.dao.UserDaoImpl; import com.ledao.diy.AnnotationPointCut; import com.ledao.service.StudentService; import com.ledao.service.UserService; import com.ledao.service.impl.StudentServiceImpl; import com.ledao.service.impl.UserServiceImpl; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration @EnableAspectJAutoProxy public class MyConfig {
@Bean public StudentService studentService(){ return new StudentServiceImpl(); }
@Bean public AnnotationPointCut annotationPointCut(){ return new AnnotationPointCut(); } }
|