介绍
JSON Server的作用是用来模拟服务端接口数据,一般用在前后端分离后,前端人员可以不依赖API开发,而在本地搭建一个JSON服务,自己产生测试数据
安装和启动
安装
使用npm安装,安装命令如下:
1
| npm install -g json-server
|
启动
要在JSON数据文件所在的文件夹打开cmd命令窗口并使用以下命令,启动命令如下所示,默认端口是3000
,使用的数据文件是db.json
(没有这个文件会自动新建,名称可以自己改掉)
1
| json-server --watch db.json
|
如果要修改端口加上--port
参数,后面接端口号,下面命令的端口号是1314
1
| json-server --watch --port 1314 db.json
|
相关启动参数如下表所示
参数 |
简写 |
默认值 |
说明 |
--config |
-c |
指定配置文件 |
[默认值: “json-server.json”] |
--port |
-p |
设置端口 [默认值: 3000] |
Number |
--host |
-H |
设置域 [默认值: “0.0.0.0”] |
String |
--watch |
-w |
Watch file(s) |
是否监听 |
--routes |
-r |
指定自定义路由 |
|
--middlewares |
-m |
指定中间件 files |
[数组] |
--static |
-s |
Set static files directory |
静态目录,类比:express的静态目录 |
--readonly |
--ro |
Allow only GET requests [布尔] |
|
--nocors |
--nc |
Disable Cross-Origin Resource Sharing [布尔] |
|
--no |
gzip |
--ng Disable GZIP Content-Encoding [布尔] |
|
--snapshots |
-S |
Set snapshots directory [默认值: “.”] |
|
--delay |
-d |
Add delay to responses (ms) |
|
--id |
-i |
Set database id property (e.g. _id) [默认值: “id”] |
|
--foreignKeySuffix |
-- |
fks Set foreign key suffix (e.g. _id as in post_id) |
[默认值: “Id”] |
--help |
-h |
显示帮助信息 |
[布尔] |
--version |
-v |
显示版本号 |
[布尔] |
测试
数据文件
要测试的数据文件db.json
内容如下,students
和classes
是复数形式,学生的班级id用classId
表示,班级的学生们的id用studentIds
表示,不然关联查询可能会有问题
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| { "students": [ { "id": "1", "name": "小明", "age": "18", "classId": "1" }, { "id": "2", "name": "小林", "age": "22", "classId": "2" }, { "id": "3", "name": "小花", "age": "21", "classId": "3" }, { "id": "4", "name": "小白", "age": "18", "classId": "1" } ], "classes": [ { "id": "1", "name": "软件工程1班", "studentIds": ["1","4"] }, { "id": "2", "name": "软件工程2班", "studentIds": ["2"] }, { "id": "3", "name": "软件工程3班", "studentIds": ["3"] } ] }
|
查询
查询使用的是GET
请求
查询全部学生
1
| http://localhost:3000/students
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| [ { "id": "1", "name": "小明", "age": "18", "classId": "1" }, { "id": "2", "name": "小林", "age": "22", "classId": "2" }, { "id": "3", "name": "小花", "age": "21", "classId": "3" }, { "id": "4", "name": "小白", "age": "18", "classId": "1" } ]
|
根据id查询学生,RestFul风格,默认是根据id查询
1
| http://localhost:3000/students/1
|
1 2 3 4 5 6
| { "id": "1", "name": "小明", "age": "18", "classId": "1" }
|
根据id查询学生,参数直接拼接在url中
1
| http://localhost:3000/students?id=2
|
1 2 3 4 5 6 7 8
| [ { "id": "2", "name": "小林", "age": "22", "classId": "2" } ]
|
分页查询,_page
是当前页,_limit
是每页数据的数量(默认是10)
1
| http://localhost:3000/students?_page=1&_limit=2
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| [ { "id": "1", "name": "小明", "age": "18", "classId": "1" }, { "id": "2", "name": "小林", "age": "22", "classId": "2" } ]
|
查询学生(排序),_sort
是要排序的字段,_order
是排序的方式(默认是升序,也就是ASC)
1
| http://localhost:3000/students?_sort=id&_order=desc
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| [ { "id": "4", "name": "小白", "age": "18", "classId": "1" }, { "id": "3", "name": "小花", "age": "21", "classId": "3" }, { "id": "2", "name": "小林", "age": "22", "classId": "2" }, { "id": "1", "name": "小明", "age": "18", "classId": "1" } ]
|
关联查询(检索上级数据),根据学生数据里的班级id检索班级信息,class
是classId
去掉Id
后的结果
1
| http://localhost:3000/students?_expand=class
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| [ { "id": "1", "name": "小明", "age": "18", "classId": "1", "class": { "id": "1", "name": "软件工程1班", "studentIds": [ "1", "4" ] } }, { "id": "2", "name": "小林", "age": "22", "classId": "2", "class": { "id": "2", "name": "软件工程2班", "studentIds": [ "2" ] } }, { "id": "3", "name": "小花", "age": "21", "classId": "3", "class": { "id": "3", "name": "软件工程3班", "studentIds": [ "3" ] } }, { "id": "4", "name": "小白", "age": "18", "classId": "1", "class": { "id": "1", "name": "软件工程1班", "studentIds": [ "1", "4" ] } } ]
|
关联查询(检索下级数据),根据班级数据里的学生id检索学生信息
1
| http://localhost:3000/classes?_embed=students
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| [ { "id": "1", "name": "软件工程1班", "studentIds": [ "1", "4" ], "students": [ { "id": "1", "name": "小明", "age": "18", "classId": "1" }, { "id": "4", "name": "小白", "age": "18", "classId": "1" } ] }, { "id": "2", "name": "软件工程2班", "studentIds": [ "2" ], "students": [ { "id": "2", "name": "小林", "age": "22", "classId": "2" } ] }, { "id": "3", "name": "软件工程3班", "studentIds": [ "3" ], "students": [ { "id": "3", "name": "小花", "age": "21", "classId": "3" } ] } ]
|
添加
添加使用的是POST
请求,要使用HTTP请求工具发送请求(我使用的是Apifox
)
参数不能直接拼接在url中要放在body中(全部参数都加上,包括id,id不要和前面重复),我这里JSON的数据类型都是String而不是Number(包括id),主要是为了关联查询不出问题
1
| http://localhost:3000/students
|
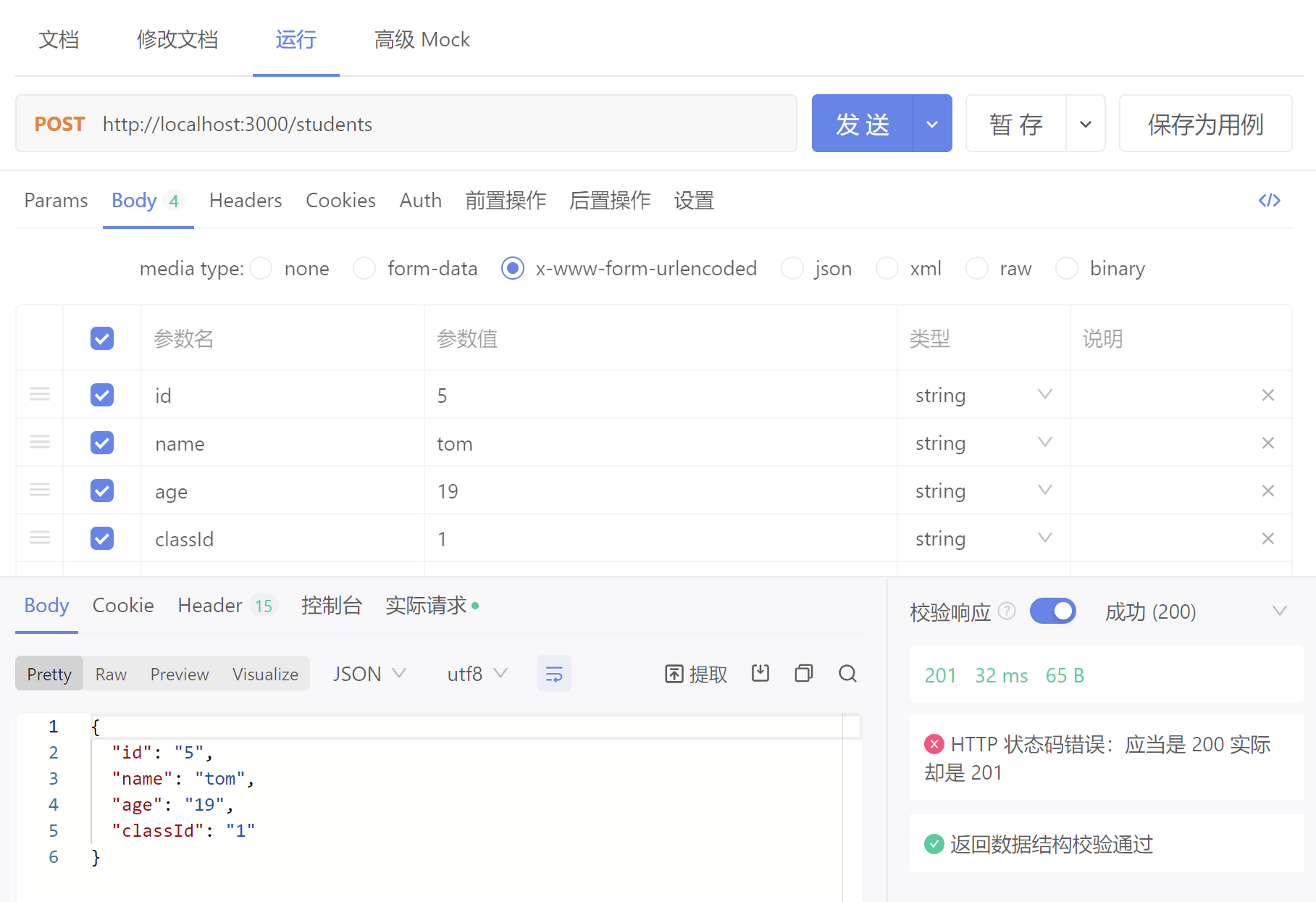
修改
修改使用的是PATCH
请求,参数不能直接拼接在url中要放在body中,但是id要放在url中(RestFul风格)
1
| http://localhost:3000/students/5
|
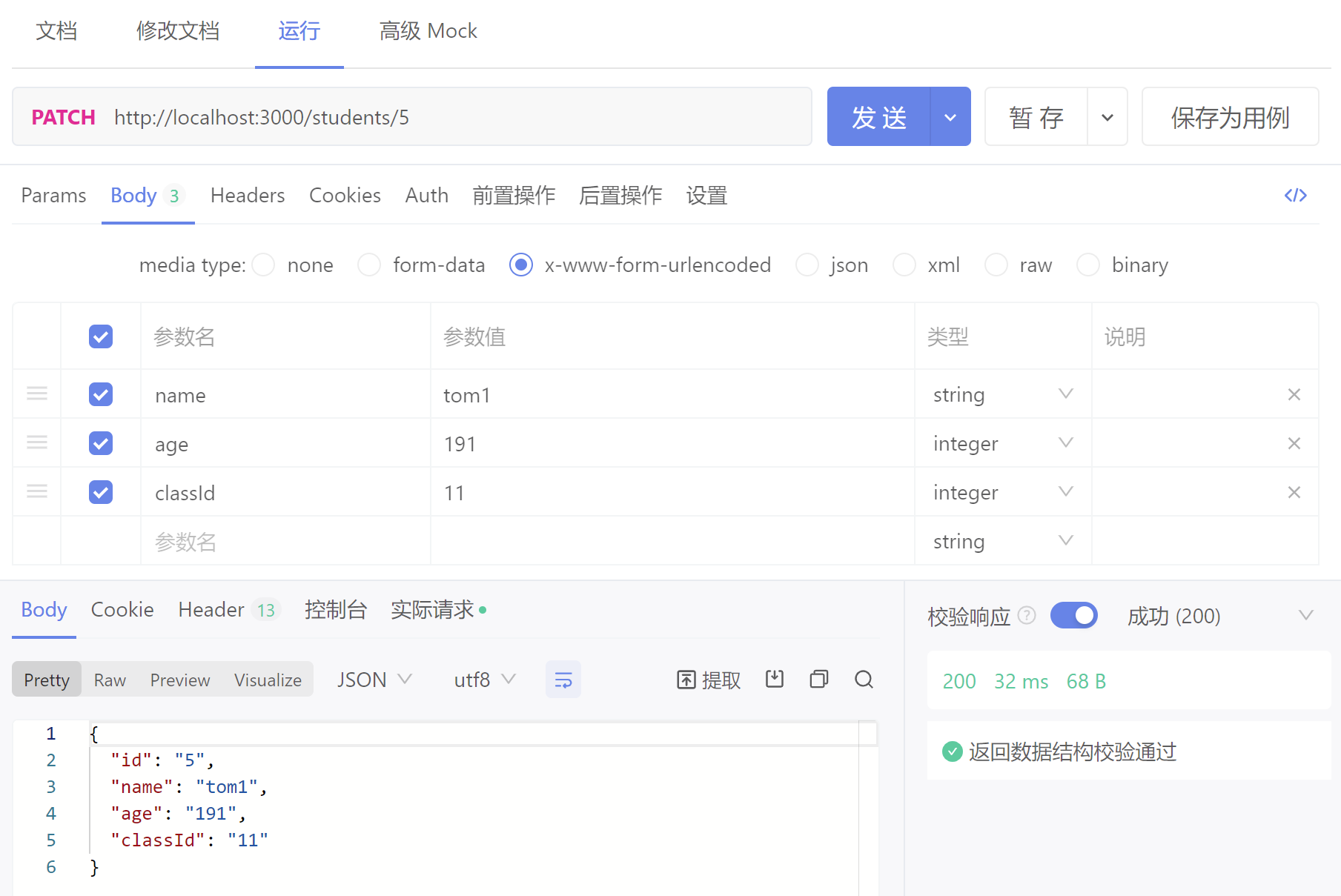
删除
修改使用的是DELETE
请求,id直接放在url中(RestFul风格)
1
| http://localhost:3000/students/5
|
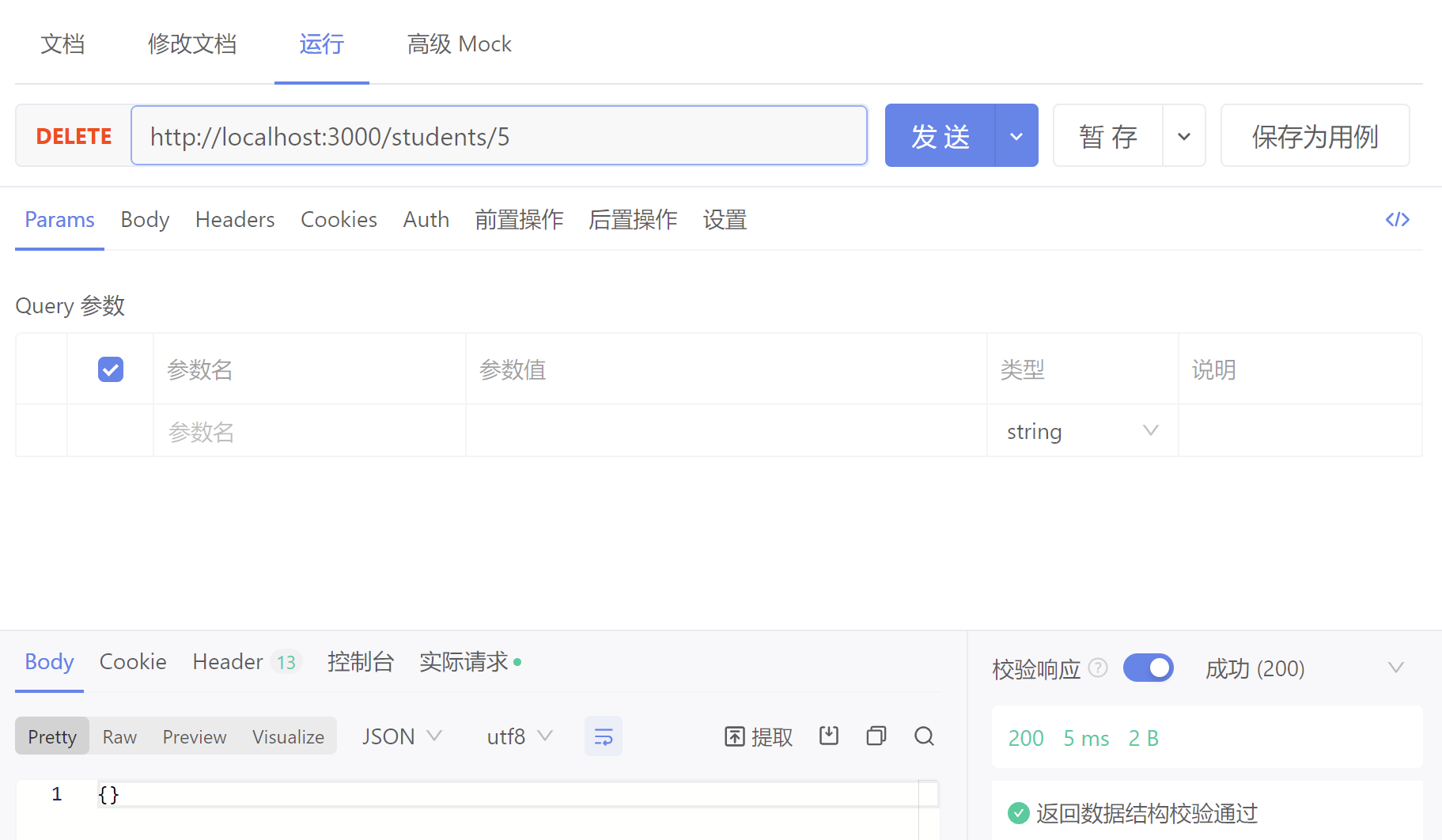