概述
Vue.js 2.0版本推荐使用Axios来完成ajax请求,Axios是一个基于Promise的HTTP库,可以用在浏览器和node.js中,推荐使用Axios
,不推荐使用vue-resource
引入
直接在页面引入
1
| <script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script>
|
使用命令安装
1
| npm install --save axios
|
get请求示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script type="text/javascript" src="js/vue.js"></script> <script src="https://cdn.staticfile.org/vue-router/2.7.0/vue-router.min.js"></script> <script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script> </head> <body> <div id="app"> <p>{{returnInfo}}</p> <p>总数量:{{getTotal}}</p> <p v-for="(value,index) in returnInfo"> 编号:{{value.id}},名称:{{value.name}},数量:{{value.num}} <button @click="addOne(index)">加1</button> <button @click="lessOne(index)">减1</button> </p> </div> <script type="text/javascript"> new Vue({ el: '#app', data() { return { returnInfo: null, total: 0 } }, mounted() { axios .get('https://www.zoutl.cn/getFruitListJson') .then(response => (this.returnInfo = response.data.rows)) .catch(function (error) { console.log(error); }); }, methods: { addOne: function (index) { this.returnInfo[index].num++; }, lessOne: function (index) { if (this.returnInfo[index].num > 0) { this.returnInfo[index].num--; } } }, computed: { getTotal: function () { let total = 0; for (let i = 0; i < this.returnInfo.length; i++) { total += this.returnInfo[i].num; } return total; } } }) </script> </body> </html>
|
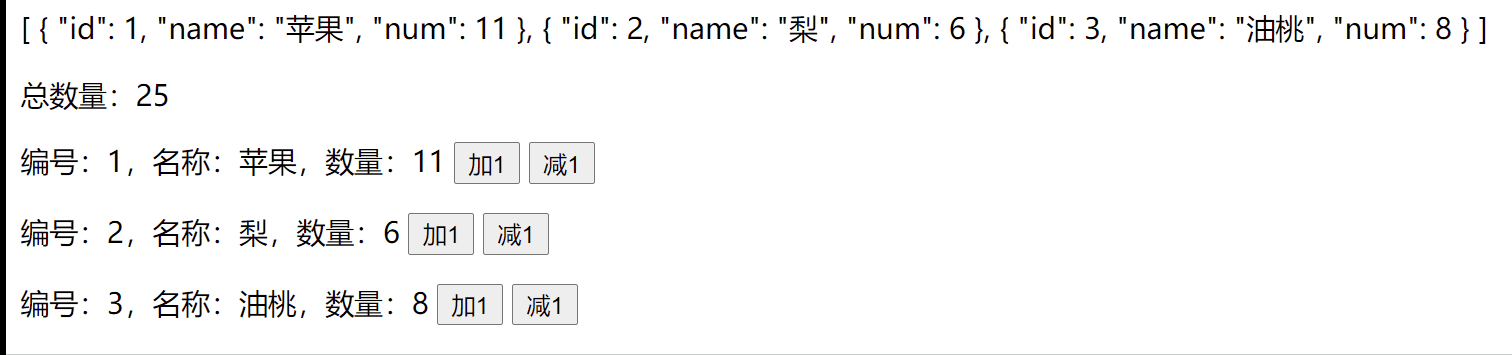
post请求示例
直接在url中拼接要传递的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script type="text/javascript" src="js/vue.js"></script> <script src="https://cdn.staticfile.org/vue-router/2.7.0/vue-router.min.js"></script> <script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script> </head> <body> <div id="app" style="margin-left: 20%;margin-top: 10%"> <p>输入水果的信息:</p> 编号:<input type="text" v-model="fruitId"/> 名称:<input type="text" v-model="fruitName"/> 数量:<input type="text" v-model="fruitNum"/> <button @click="submitData">提交</button> </div> <script type="text/javascript"> new Vue({ el: '#app', data: { fruitId: 1, fruitName: '苹果', fruitNum: 3 }, methods: { submitData: function () { axios .post('https://www.zoutl.cn/getFruitJson2?id=' + this.fruitId + '&name=' + this.fruitName + '&num=' + this.fruitNum + '') .then(function (response) { let id = response.data.id; let name = response.data.name; let num = response.data.num; alert("编号:" + id + ",名称:" + name + ",数量:" + num); }) .catch(function (error) { console.log(error); }); } } }) </script> </body> </html>
|
使用URLSearchParams传递数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script type="text/javascript" src="js/vue.js"></script> <script src="https://cdn.staticfile.org/vue-router/2.7.0/vue-router.min.js"></script> <script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script> </head> <body> <div id="app" style="margin-left: 20%;margin-top: 10%"> <p>输入水果的信息:</p> 编号:<input type="text" v-model="fruitId"/> 名称:<input type="text" v-model="fruitName"/> 数量:<input type="text" v-model="fruitNum"/> <button @click="submitData">提交</button> </div> <script type="text/javascript"> new Vue({ el: '#app', data: { fruitId: 1, fruitName: '苹果', fruitNum: 3, myInfo: '' }, methods: { submitData: function () { let param = new URLSearchParams(); param.append("id", this.fruitId); param.append("name", this.fruitName); param.append("num", this.fruitNum); axios .post('https://www.zoutl.cn/getFruitJson2', param) .then(function (response) { let id = response.data.id; let name = response.data.name; let num = response.data.num; alert("编号:" + id + ",名称:" + name + ",数量:" + num); }) .catch(function (error) { console.log(error); }); } } }) </script> </body> </html>
|
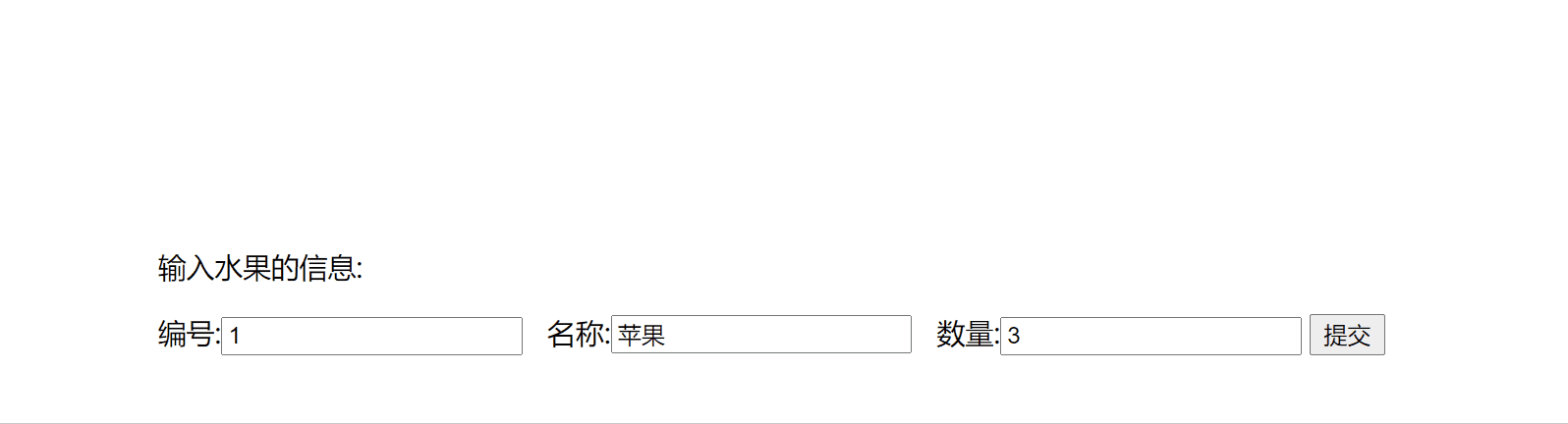