GitHub完整代码地址:https://github.com/a6678696/MyBatisPageHelperTest
pom.xml引入分页插件
1 2 3 4 5
| <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper-spring-boot-starter</artifactId> <version>1.3.0</version> </dependency>
|
application.yml配置
1 2 3 4 5
| pagehelper: helperDialect: mysql reasonable: true supportMethodsArguments: true params: count=countSql
|
User.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package com.ledao.entity;
import lombok.Data;
@Data public class User {
private Integer id;
private String userName;
private String password;
private String nickName; }
|
UserMapper.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package com.ledao.mapper;
import com.ledao.entity.User;
import java.util.List;
public interface UserMapper {
List<User> findAll(); }
|
UserMapper.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.ledao.mapper.UserMapper"> <resultMap id="UserResult" type="User"> <result property="id" column="id"/> <result property="userName" column="userName"/> <result property="password" column="password"/> <result property="nickName" column="nickName"/> </resultMap>
<select id="findAll" resultMap="UserResult"> select * from t_user </select> </mapper>
|
UserService.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package com.ledao.service;
import com.github.pagehelper.PageInfo;
public interface UserService {
PageInfo findAll(int page, int size); }
|
UserServiceImpl.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package com.ledao.service.impl;
import com.github.pagehelper.PageHelper; import com.github.pagehelper.PageInfo; import com.ledao.entity.User; import com.ledao.mapper.UserMapper; import com.ledao.service.UserService; import org.springframework.stereotype.Service;
import javax.annotation.Resource; import java.util.List;
@Service("userService") public class UserServiceImpl implements UserService {
@Resource private UserMapper userMapper;
@Override public PageInfo findAll(int page, int size) { PageHelper.startPage(page, size); List<User> userList = userMapper.findAll(); PageInfo result = new PageInfo<>(userList); return result; } }
|
UserController.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.ledao.controller;
import com.github.pagehelper.PageInfo; import com.ledao.service.UserService; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
@RestController @RequestMapping("/user") public class UserController {
@Resource private UserService userService;
@RequestMapping("/findAll") public PageInfo findAll(int page, int size) { return userService.findAll(page, size); } }
|
结果
浏览器地址栏输入http://localhost/user/findAll?page=3&size=4
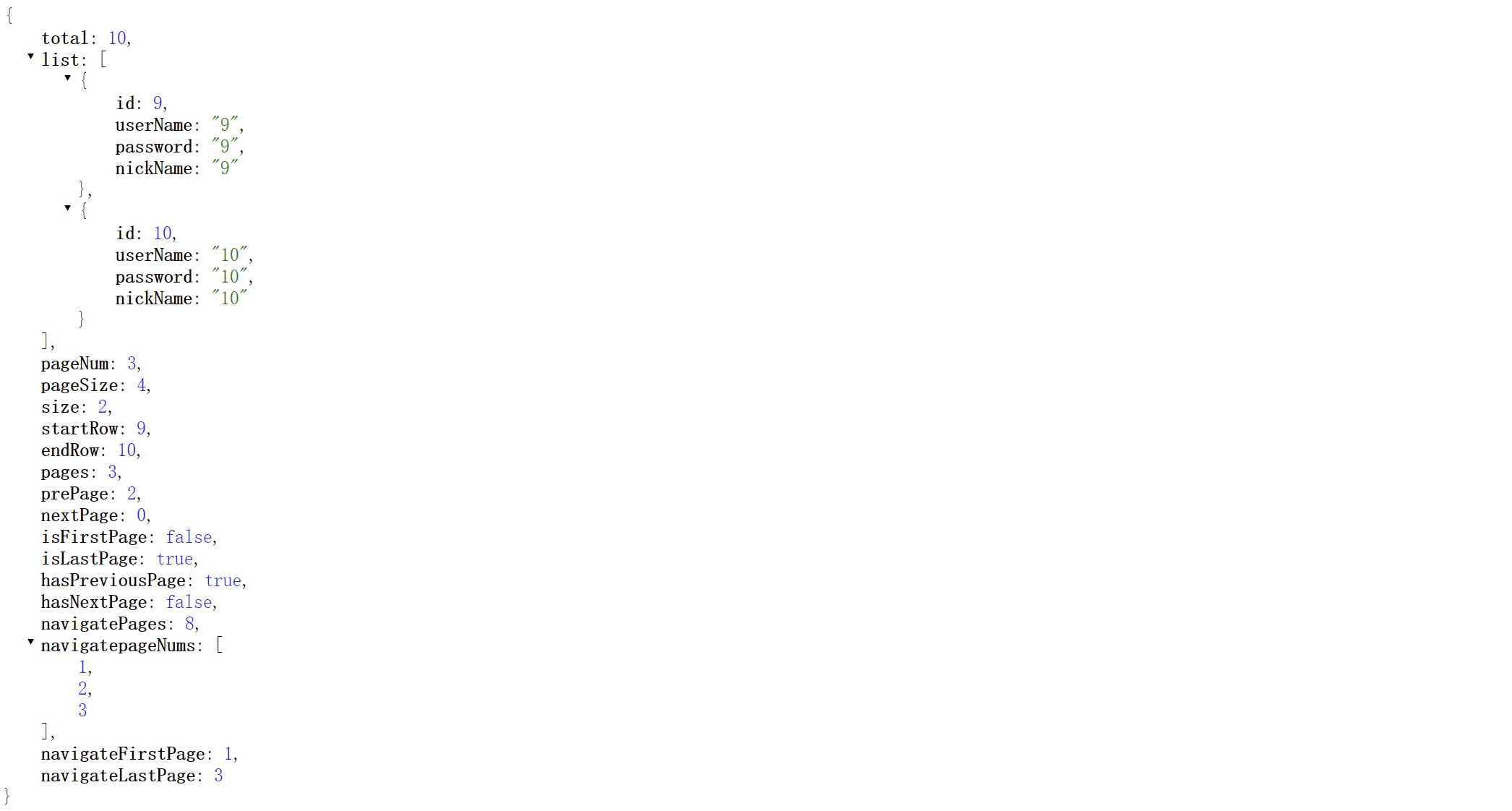