引入依赖
1 2 3 4 5
| <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.1</version> </dependency>
|
application.yml配置
1 2 3 4 5 6 7 8
| mybatis: mapper-locations: classpath:mybatis/mapper/*.xml type-aliases-package: com.ledao.entity configuration: map-underscore-to-camel-case: true
|
加入@MapperScan注解
在项目的启动类中加入@MapperScan
注解,这样就可以指定要扫描的Mapper类的包的路径了
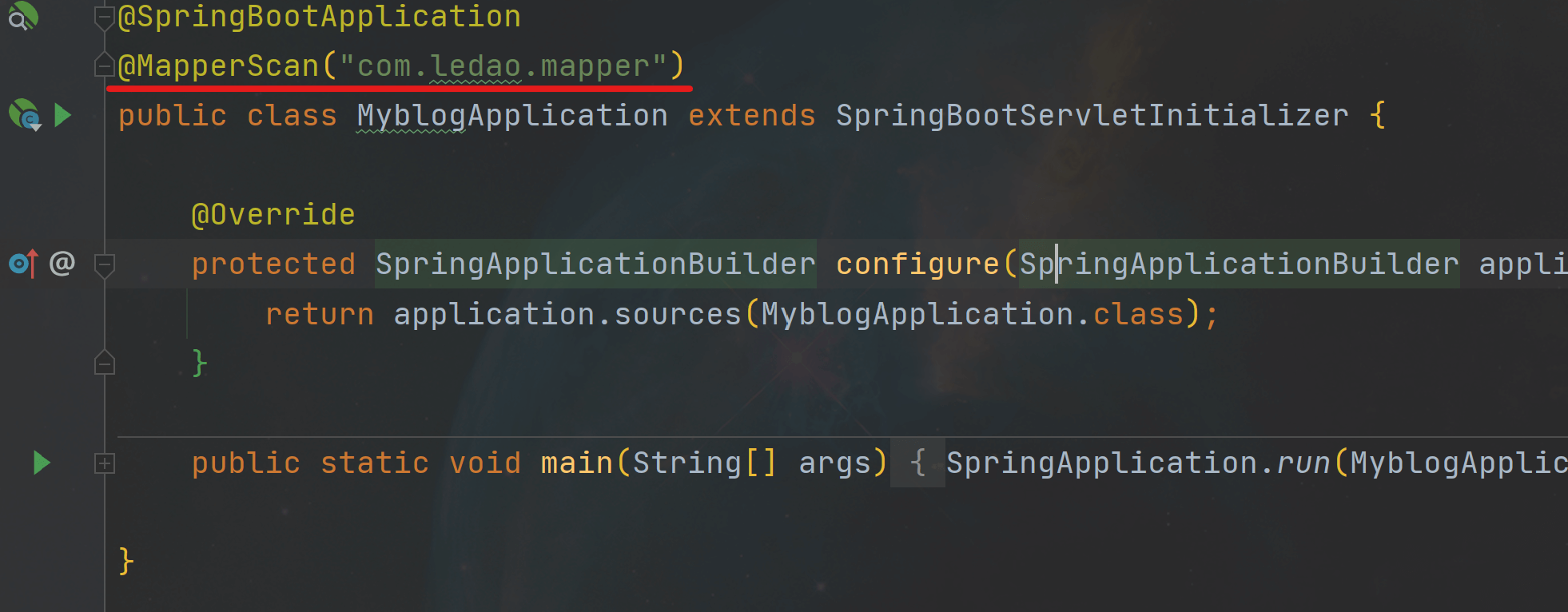
项目代码示例
以对一个博客类进行操作为例
Blog类
已省略setter和getter方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78
| package com.ledao.entity; import java.util.Date;
public class Blog {
private Integer id;
private String title;
private String summary;
private String content;
private Date releaseDate;
private Integer click;
private Integer blogTypeId;
private BlogType blogType;
private String imageName;
private Integer blogCount;
private String releaseDateStr;
private Integer blogNum;
private Integer isLike;
private Integer likeNum;
private Integer isMenuBlog;
private Date setMenuBlogDate; }
|
BlogMapper接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| package com.ledao.mapper; import com.ledao.entity.Blog; import java.util.List; import java.util.Map;
public interface BlogMapper {
List<Blog> list(Map map);
Long getCount(Map map);
Integer add(Blog blog);
Integer update(Blog blog);
Integer delete(Integer id);
Blog findById(Integer id);
List<Blog> countList();
List<Blog> findByBlogTypeId(Integer blogTypeId);
Blog getPreviousBlog(Integer id);
Blog getNextBlog(Integer id);
List<Blog> getMenuBlogList(); }
|
BlogMapper的XML文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142
| <?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.ledao.mapper.BlogMapper"> <resultMap id="BlogResult" type="Blog"> <result property="id" column="id"/> <result property="title" column="title"/> <result property="summary" column="summary"/> <result property="releaseDate" column="releaseDate"/> <result property="click" column="click"/> <result property="content" column="content"/> <result property="blogTypeId" column="blogTypeId"/> <result property="isMenuBlog" column="isMenuBlog"/> <result property="setMenuBlogDate" column="setMenuBlogDate"/> </resultMap> <select id="list" parameterType="map" resultMap="BlogResult"> select * from t_blog <where> <if test="title != null and title != ''"> and title like #{title} </if> <if test="blogTypeId != null"> and blogTypeId = #{blogTypeId} </if> <if test="isMenuBlog != null"> and isMenuBlog = #{isMenuBlog} </if> <if test="isMenuBlogKey != null"> and isMenuBlog != 1 </if> <if test="releaseDateStr != null and releaseDateStr != ''"> and date_format(releaseDate, '%Y年%m月') = #{releaseDateStr} </if> </where> <if test="sortByReleaseDate == null"> order by releaseDate desc </if> <if test="start != null and size != null"> limit #{start},#{size} </if> </select> <select id="getCount" parameterType="map" resultType="java.lang.Long"> select count(*) from t_blog <where> <if test="title != null and title != ''"> and title like #{title} </if> <if test="blogTypeId != null"> and blogTypeId = #{blogTypeId} </if> <if test="isMenuBlog != null"> and isMenuBlog = #{isMenuBlog} </if> <if test="isMenuBlogKey != null"> and isMenuBlog != 1 </if> <if test="releaseDateStr != null and releaseDateStr != ''"> and date_format(releaseDate, '%Y年%m月') = #{releaseDateStr} </if> </where> </select> <insert id="add" parameterType="Blog"> insert into t_blog (title, summary, content, releaseDate, click, blogTypeId,isMenuBlog) values (#{title}, #{summary}, #{content}, now(), 0, #{blogTypeId},0); </insert> <update id="update" parameterType="Blog"> update t_blog <set> <if test="title != null and title != ''"> title=#{title}, </if> <if test="summary != null and summary != ''"> summary=#{summary}, </if> <if test="content != null and content != ''"> content=#{content}, </if> <if test="click != null"> click=#{click}, </if> <if test="blogTypeId != null"> blogTypeId=#{blogTypeId}, </if> <if test="isMenuBlog != null"> isMenuBlog = #{isMenuBlog}, </if> <if test="setMenuBlogDate != null"> setMenuBlogDate=now(), </if> </set> where id = #{id} </update> <delete id="delete" parameterType="integer"> delete from t_blog where id = #{id} </delete> <select id="findById" parameterType="integer" resultMap="BlogResult"> select * from t_blog where id = #{id} </select> <select id="countList" resultMap="BlogResult"> select date_format(releaseDate, '%Y年%m月') as releaseDateStr, count(*) as blogCount from t_blog where isMenuBlog=0 group by date_format(releaseDate, '%Y年%m月') order by date_format(releaseDate, '%Y年%m月') desc </select> <select id="findByBlogTypeId" parameterType="integer" resultMap="BlogResult"> select * from t_blog where blogTypeId = #{blogTypeId} order by releaseDate desc </select> <select id="getPreviousBlog" parameterType="integer" resultType="com.ledao.entity.Blog"> SELECT * FROM t_blog WHERE id < #{id} ORDER BY id DESC LIMIT 1; </select> <select id="getNextBlog" parameterType="integer" resultType="com.ledao.entity.Blog"> SELECT * FROM t_blog WHERE id > #{id} ORDER BY id asc LIMIT 1; </select> <select id="getMenuBlogList" resultMap="BlogResult"> select * from t_blog where isMenuBlog = 1 order by setMenuBlogDate asc </select> </mapper>
|
BlogService接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| package com.ledao.service; import com.ledao.entity.Blog; import java.util.List; import java.util.Map;
public interface BlogService {
List<Blog> list(Map map);
Long getCount(Map map);
Integer add(Blog blog);
Integer update(Blog blog);
Integer delete(Integer id);
Blog findById(Integer id);
List<Blog> countList();
List<Blog> findByBlogTypeId(Integer blogTypeId);
Blog getPreviousBlog(Integer id);
Blog getNextBlog(Integer id);
List<Blog> getMenuBlogList(); }
|
BlogService接口的实现类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79
| package com.ledao.service.impl; import com.ledao.entity.Blog; import com.ledao.mapper.BlogMapper; import com.ledao.service.BlogService; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; import java.util.Map;
@Service("blogService") public class BlogServiceImpl implements BlogService { @Resource private BlogMapper blogMapper; @Override public List<Blog> list(Map map) { return blogMapper.list(map); } @Override public Long getCount(Map map) { return blogMapper.getCount(map); } @Override public Integer add(Blog blog) { return blogMapper.add(blog); } @Override public Integer update(Blog blog) { return blogMapper.update(blog); } @Override public Integer delete(Integer id) { return blogMapper.delete(id); } @Override public Blog findById(Integer id) { return blogMapper.findById(id); } @Override public List<Blog> countList() { return blogMapper.countList(); } @Override public List<Blog> findByBlogTypeId(Integer blogTypeId) { return blogMapper.findByBlogTypeId(blogTypeId); } @Override public Blog getPreviousBlog(Integer id) { return blogMapper.getPreviousBlog(id); } @Override public Blog getNextBlog(Integer id) { return blogMapper.getNextBlog(id); } @Override public List<Blog> getMenuBlogList() { return blogMapper.getMenuBlogList(); } }
|