前端向后端发送的数据有当前页、每页记录数,后端向前端发送的数据有查询到的数据集合、数据总记录数(满足查询条件的全部数据的总记录数)
前端分页代码
1 2 3 4 5 6 7 8 9 10
| <el-pagination align="center" style="margin-top: 8px" background layout="prev, pager, next" :current-page.sync="pagination.page" :page-size.sync="pagination.size" :total="pagination.count" @current-change="handleCurrentChange"> </el-pagination>
|
分页属性
包括当前页,每页记录数,数据总记录数
1 2 3 4 5
| pagination: { page: 1, size: 2, count: 0, }
|
更新当前页的值
1 2 3 4
| handleCurrentChange(page) { this.pagination.page = page; }
|
请求后台
1 2 3 4 5 6
| axios .get('http://localhost/paper/getListFindByUserId?userId=' + sessionStorage.getItem("userId") + '&page=' + this.pagination.page + '&size=' + this.pagination.size) .then(response => (this.myPaper = response.data.rows, this.pagination.count = response.data.total)) .catch(function (error) { console.log(error); });
|
mapper.xml代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <select id="list" parameterType="map" resultMap="paperResult"> select * from t_paper <where> <if test="userId != null"> and userId = #{userId} </if> </where> order by id desc <if test="start != null and size != null"> limit #{start},#{size} </if> </select> <select id="getCount" parameterType="map" resultType="java.lang.Long"> select count(*) from t_paper <where> <if test="userId != null"> and userId = #{userId} </if> </where> </select>
|
请求的后端方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
|
@RequestMapping("/getListFindByUserId") public Map getListFindByUserId(Integer userId, @RequestParam(value = "page",required = false)Integer page, @RequestParam(value = "size",required = false)Integer size) { PageBean pageBean = new PageBean(page, size); Map resultMap = new HashMap<>(16); Map map = new HashMap<>(16); map.put("userId", userId); map.put("start", pageBean.getStart()); map.put("size", pageBean.getPageSize()); List<Paper> paperList = paperService.list(map); resultMap.put("total", paperService.getCount(map)); resultMap.put("rows", paperList); return resultMap; }
|
分页Model类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
public class PageBean {
private int page;
private int pageSize;
private int start;
public PageBean(int page, int pageSize) { super(); this.page = page; this.pageSize = pageSize; }
public int getPage() { return page; }
public void setPage(int page) { this.page = page; }
public int getPageSize() { return pageSize; }
public void setPageSize(int pageSize) { this.pageSize = pageSize; }
public int getStart() { return (page - 1) * pageSize; } }
|
结果
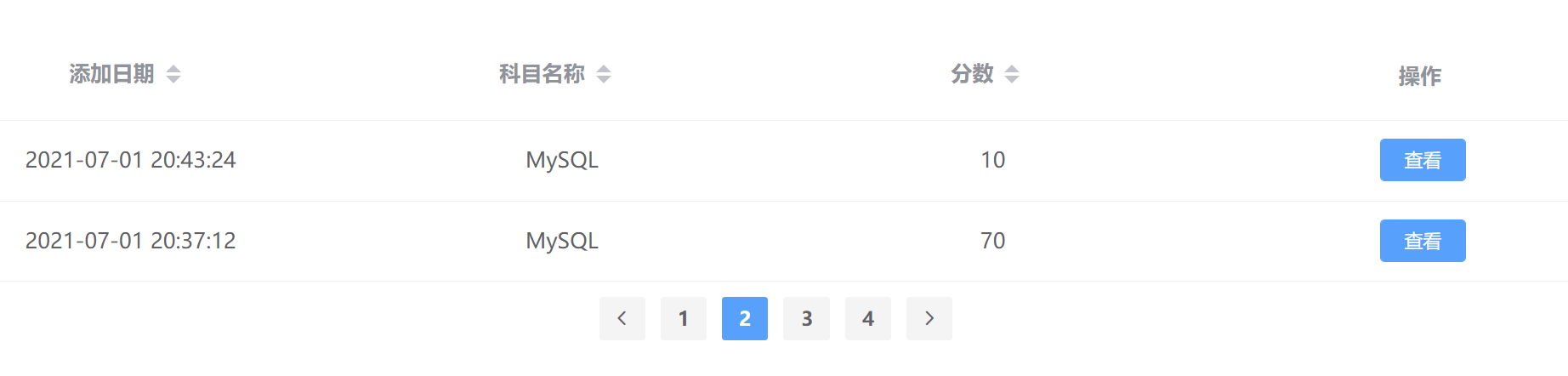