前提
已经搭建好RabbitMQ基本环境,搭建步骤查看博客:搭建RabbitMQ基本环境
配置好RabbitMQ的交换机、队列、队列绑定交换机,具体步骤查看博客:RabbitMQ实现生产者发送消息 ,rabbitmq-common模块的RabbitMQConfig.java就是配置类
项目结构
实现过程
application.yml配置
添加一个application.yml配置文件,内容如下:
1 2 3 4 5 6 7 8 9 10
| server: port: 81
spring: rabbitmq: host: 192.168.0.145 port: 5672 username: admin password: admin virtual-host: /
|
接收消息页面
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>接收消息</title> <script src="https://cdn.staticfile.org/jquery/2.1.1/jquery.min.js"></script> <script> function receiveMessage() { $.ajax({ url: "/receiveMessage", type: "get", success: function (result) { $("#messageReceive").html(result.message); }, }); } </script> </head> <body> <div> 接收的消息:<span style="color: red" id="messageReceive">看这里</span><br> <a href="javascript:receiveMessage()"> <button>接收</button> </a> </div> </body> </html>
|
消费者Service接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.ledao.consumer.service;
public interface RabbitMQConsumerService {
String receiveMessage(); }
|
Service接口实现类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.ledao.consumer.service.impl;
import com.ledao.common.config.RabbitMQConfig; import com.ledao.consumer.service.RabbitMQConsumerService; import org.springframework.amqp.core.AmqpTemplate; import org.springframework.amqp.rabbit.annotation.RabbitListener; import org.springframework.stereotype.Service;
import javax.annotation.Resource;
@Service("rabbitMQConsumerService") public class RabbitMQConsumerServiceImpl implements RabbitMQConsumerService {
@Resource private AmqpTemplate amqpTemplate;
@Override public String receiveMessage() { return (String) amqpTemplate.receiveAndConvert(RabbitMQConfig.DIRECT_QUEUE); } }
|
IndexController.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| package com.ledao.consumer.controller;
import com.ledao.consumer.service.RabbitMQConsumerService; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView;
import javax.annotation.Resource; import java.util.Date; import java.util.HashMap; import java.util.Map;
@Controller public class IndexController {
@Resource private RabbitMQConsumerService rabbitMQConsumerService;
@RequestMapping("/toReceiveMessagePage") public ModelAndView toReceiveMessagePage() { ModelAndView mav = new ModelAndView(); mav.setViewName("receiveMessagePage"); return mav; }
@ResponseBody @RequestMapping("/receiveMessage") public Map<String, Object> receiveMessage() { Map<String, Object> resultMap = new HashMap<>(16); String message = rabbitMQConsumerService.receiveMessage(); resultMap.put("message", message != null ? message : "消息队列为空!!"+new Date()); return resultMap; } }
|
测试
浏览器地址栏输入:http://localhost:81/toReceiveMessagePage 打开接收消息页面,然后点击接收按钮即可
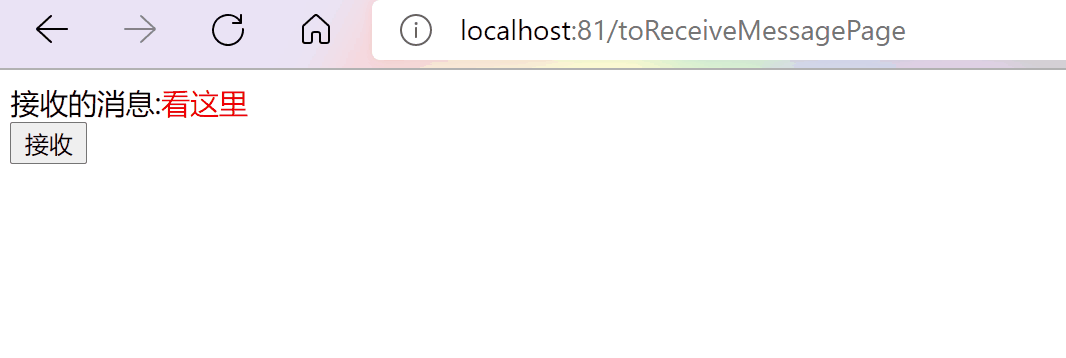
PS.
本博客只是实现了手动接收队列消息,监听队列接收消息查看博客:RabbitMQ实现监听队列接收消息