引入依赖
1 2 3 4 5 6 7 8 9 10
| <dependency> <groupId>com.baomidou</groupId> <artifactId>mybatis-plus-boot-starter</artifactId> <version>3.3.2</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.1.10</version> </dependency>
|
application.yml配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| server: port: 8080 servlet: context-path: / tomcat: uri-encoding: UTF-8
spring: servlet: multipart: enabled: true file-size-threshold: 0 max-file-size: 10MB max-request-size: 100MB datasource: type: com.alibaba.druid.pool.DruidDataSource driver-class-name: com.mysql.cj.jdbc.Driver url: jdbc:mysql://localhost:3306/db_test?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf8 username: root password: 123456 jackson: time-zone: GMT+8 date-format: yyyy-MM-dd HH:mm:ss mybatis-plus: global-config: db-config: id-type: auto mapper-locations: classpath:/mapper/**.xml
|
添加注解
启动类里也需要加一个mapper扫描配置@MapperScan(“com.ledao.mapper”)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package com.ledao;
import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication;
@MapperScan("com.ledao.mapper") @SpringBootApplication public class MyBatisPlusDemoApplication {
public static void main(String[] args) { SpringApplication.run(MyBatisPlusDemoApplication.class, args); }
}
|
测试
表结构
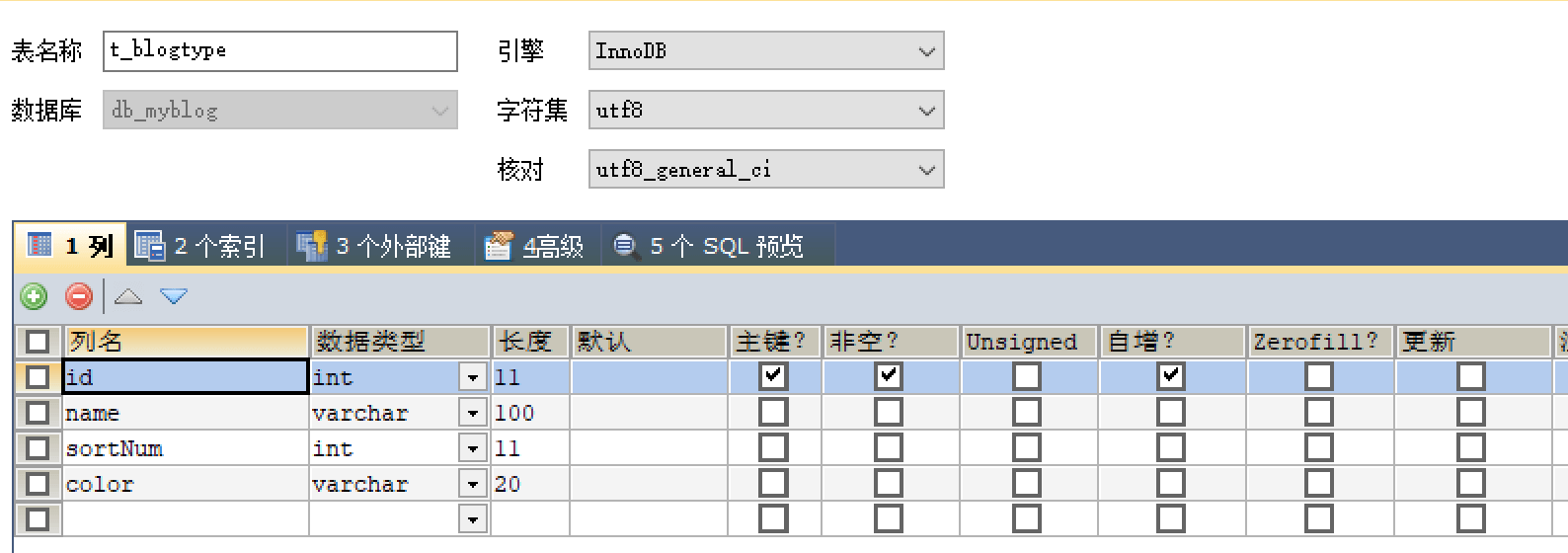
BlogType实体类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package com.ledao.entity;
import com.baomidou.mybatisplus.annotation.TableField; import com.baomidou.mybatisplus.annotation.TableId; import com.baomidou.mybatisplus.annotation.TableName; import lombok.Data;
@Data @TableName(value = "t_blogtype") public class BlogType {
@TableId private Integer id; private String name; @TableField(value = "sortNum") private Integer sortNum; }
|
BlogTypeMapper
1 2 3 4 5 6 7 8 9 10 11 12
| package com.ledao.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper; import com.ledao.entity.BlogType;
public interface BlogTypeMapper extends BaseMapper<BlogType> { }
|
测试代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.ledao;
import com.ledao.entity.BlogType; import com.ledao.mapper.BlogTypeMapper; import org.junit.jupiter.api.Test; import org.springframework.boot.test.context.SpringBootTest;
import javax.annotation.Resource; import java.util.List;
@SpringBootTest class MyBatisPlusDemoApplicationTests {
@Resource private BlogTypeMapper blogTypeMapper;
@Test void contextLoads() { List<BlogType> blogTypeList = blogTypeMapper.selectList(null); for (BlogType blogType : blogTypeList) { System.out.println(blogType.getId() + "," + blogType.getName() + "," + blogType.getSortNum()); } }
}
|
结果
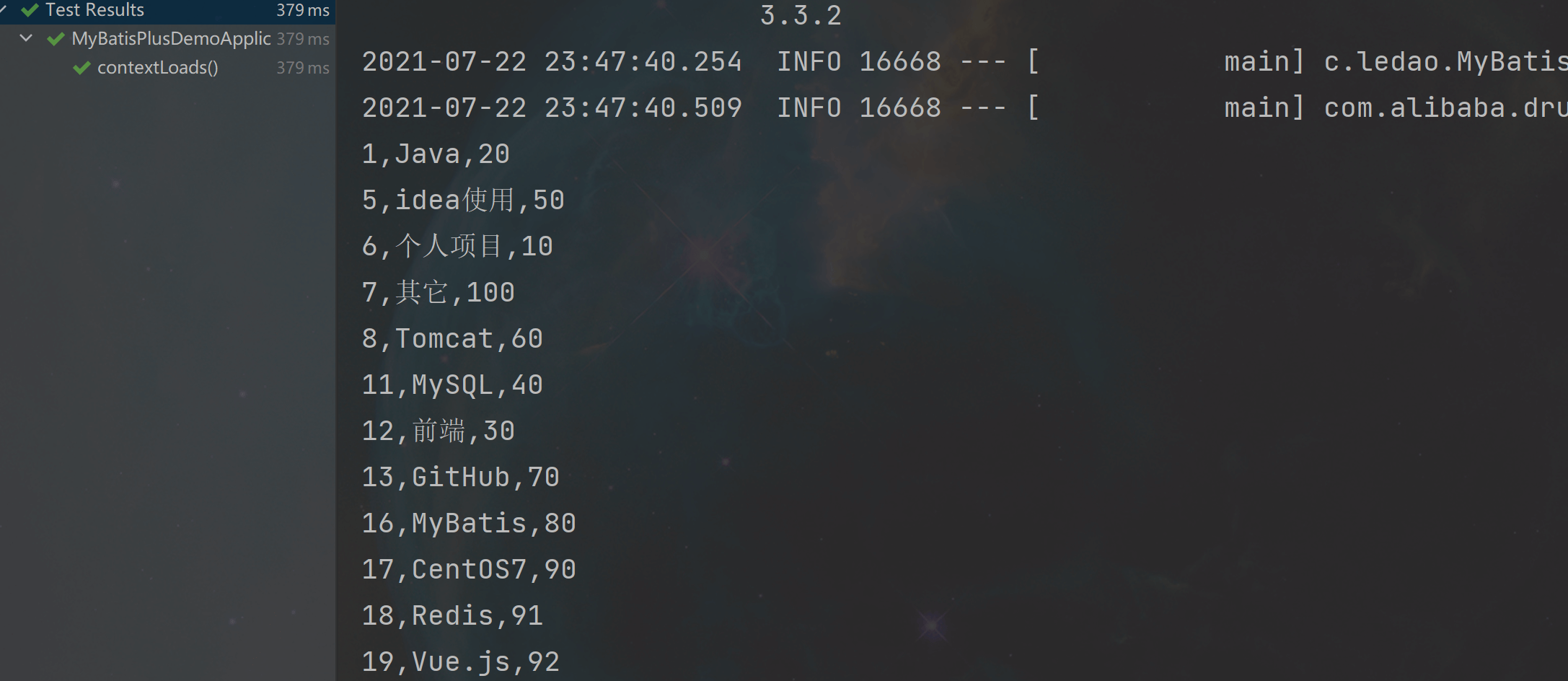