概述
微信小程序可以通过wx.request
函数发起HTTPS网络请求,从Java后端接口动态获取数据,官方文档链接为:RequestTask | 微信开放文档 (qq.com)
实现过程
跳过域名检测
使用localhost
或其他开发环境接口时,控制台报错,我们需要跳过服务器域名的校验
Java代码
新建Spring Boot项目
新建项目时,最少要添加一个Spring Web
依赖
application.yml配置文件
1 2 3 4 5 6
| server: port: 80 servlet: context-path: / tomcat: uri-encoding: utf-8
|
HelloWorldController.java
下面接口可以通过localhost/helloWorld进行访问
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package com.ledao.controller;
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class HelloWorldController {
@GetMapping("/helloWorld") public String helloWord(Integer id) { return "HelloWorld " + id; } }
|
小程序代码
页面wxml代码
1 2 3 4 5 6 7 8
| <text>结果: <text style="color: red;">{{result}}</text></text> <view> <input type="text" style="border: 2px solid red;" model:value="{{id}}" /> </view> <view style="text-align: center;margin-top: 14rpx;"> <button catchtap="submit" size="mini">提交</button> </view>
|
页面js代码
只展示了关键代码,默认是GET请求
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| Page({
data: { id: 1, result: '我是默认值' },
submit() { wx.request({ url: 'http://localhost/helloWorld', data: { id: this.data.id }, success(res) { this.setData({ result: res.data }) } }) } })
|
效果
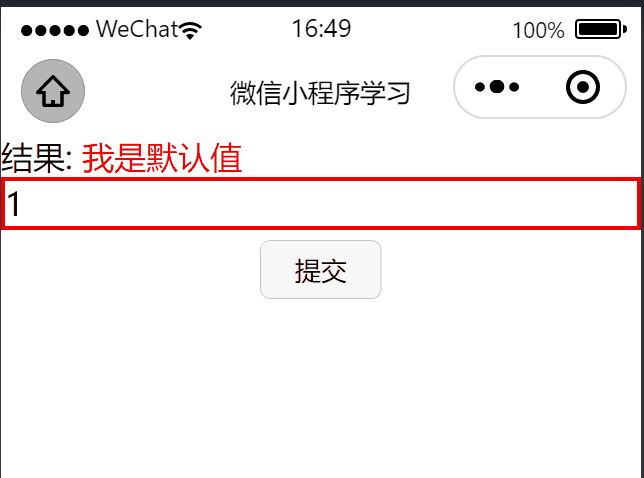
PS.
如果是POST请求需要加上请求头'content-type': 'application/x-www-form-urlencoded'
,不然无法传递参数
1 2 3
| header: { 'content-type': 'application/x-www-form-urlencoded', }
|